Java generic method: Find index of target element in list
Write a Java program to create a generic method that takes a list of any type and a target element. It returns the index of the first occurrence of the target element in the list. Return -1 if the target element cannot be found.
Sample Solution:
Java Code:
// FindElement.java
// FindElement Class
import java.util.List;
public class FindElement {
public static < T > int findIndexOfElement(List < T > list, T target) {
for (int i = 0; i < list.size(); i++) {
if (list.get(i).equals(target)) {
return i;
}
}
return -1;
}
public static void main(String[] args) {
List < Integer > numbers = List.of(1, 2, 3, 4, 5);
List < String > colors = List.of("Red", "Green", "Blue", "Orange", "White");
System.out.println("Original list elements:");
System.out.println("Nums: " + numbers);
System.out.println("Colors: " + colors);
int index1 = findIndexOfElement(numbers, 3);
System.out.println("\nIndex of 3 in numbers: " + index1); // Output: 2
int index2 = findIndexOfElement(numbers, 6);
System.out.println("Index of 6 in numbers: " + index2); // Output: -1
int index3 = findIndexOfElement(colors, "Green");
System.out.println("Index of \"Green\" in colors: " + index3); // Output: 1
int index4 = findIndexOfElement(colors, "Black");
System.out.println("Index of \"Black\" in colors: " + index4); // Output: -1
}
}
Sample Output:
Original list elements: Nums: [1, 2, 3, 4, 5] Colors: [Red, Green, Blue, Orange, White] Index of 3 in numbers: 2 Index of 6 in numbers: -1 Index of "Green" in colors: 1 Index of "Black" in colors: -1
Explanation:
In the above exercise, we define a generic method findIndexOfElement() that takes a list list and a target element target as input. With a for loop, the method iterates over the list and checks if each element is equal to the target element. If a match is found, the method returns the index of the first occurrence. If the target element cannot be found, the method returns -1.
In the main() method, we demonstrate the findIndexOfElement() method by passing a list of integers (numbers) and a list of strings (words). We find the index of specific elements in the list and print the results.
Flowchart:
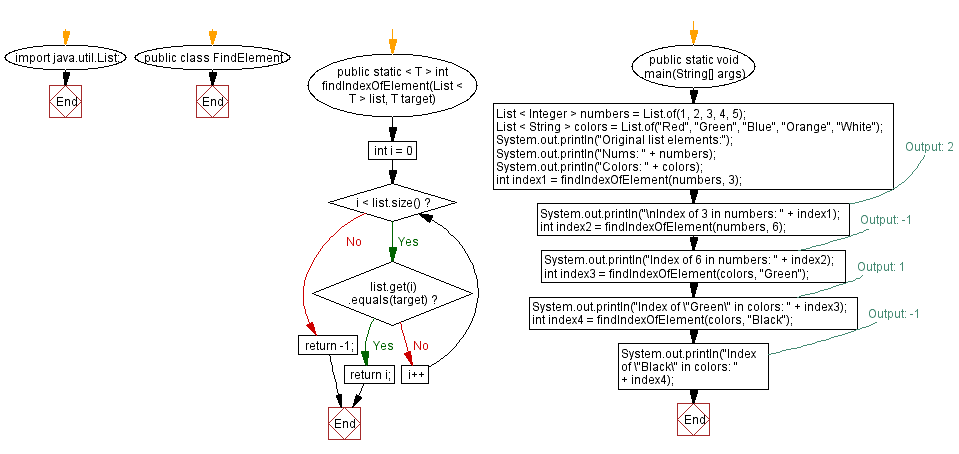
For more Practice: Solve these Related Problems:
- Write a Java program to create a generic method that returns all indices at which a target element occurs in a list.
- Write a Java program to create a generic method that finds the last index of a target element in a list using reverse iteration.
- Write a Java program to create a generic method that employs binary search to return the index of a target element in a sorted list.
- Write a Java program to create a generic method that uses recursion to locate the first occurrence of a target element in a list.
Java Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Calculate sum of even and odd numbers.
Next: Reverse list elements.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.