Java Encapsulation: Implementing an Employee Class with Getter and Setter Methods
Java Encapsulatiion: Exercise-4 with Solution
Write a Java program to create a class called Employee with private instance variables employee_id, employee_name, and employee_salary. Provide public getter and setter methods to access and modify the id and name variables, but provide a getter method for the salary variable that returns a formatted string.
Sample Solution:
Java Code:
// Employee.java
// Employee Class
class Employee {
private int employee_id;
private String employee_name;
private double employee_salary;
public int getEmployeeId() {
return employee_id;
}
public void setEmployeeId(int employeeId) {
this.employee_id = employeeId;
}
public String getEmployeeName() {
return employee_name;
}
public void setEmployeeName(String employeeName) {
this.employee_name = employeeName;
}
public double getEmployeeSalary() {
return employee_salary;
}
public void setEmployeeSalary(double employeeSalary) {
this.employee_salary = employeeSalary;
}
public String getFormattedSalary() {
return String.format("$%.2f", employee_salary);
}
}
Explanation:
In the aboove code, the Employee class encapsulates the private instance variables employee_id, employee_name, and employee_salary. The getEmployeeId() and getEmployeeName() methods are public getter methods that allow other classes to access employee_id and employee_name values, respectively. The setEmployeeId() and setEmployeeName() methods are public setter methods that allow other classes to modify employee_id and employee_name values.
The getEmployeeSalary() method is another public getter method that returns employee_salary. However, we have also added an additional method getFormattedSalary() that returns a formatted string representation of the salary. This method provides a formatted version of the salary value, adding a dollar sign and two decimal places.
// Main.java
// Main Class
public class Main {
public static void main(String[] args) {
// Create an instance of Employee
Employee employee = new Employee();
// Set values using setter methods
employee.setEmployeeId(15);
employee.setEmployeeName("Caelius Dathan");
employee.setEmployeeSalary(4900.0);
// Get values using getter methods
int employeeId = employee.getEmployeeId();
String employeeName = employee.getEmployeeName();
String formattedSalary = employee.getFormattedSalary();
// Print the values
System.out.println("Employee Details:");
System.out.println("ID: " + employeeId);
System.out.println("Name: " + employeeName);
System.out.println("Salary: " + formattedSalary);
}
}
In the Main class, an employee object within the Employee class is created. The setter methods (setEmployeeId(), setEmployeeName(), and setEmployeeSalary()) are used to set employee_id, employee_name, and employee_salary, respectively. The getter methods (getEmployeeId(), getEmployeeName(), and getFormattedSalary()) are used to retrieve employee_id, employee_name, and formatted salary, respectively.
Finally, System.out.println() statements print employee_id, employee_name, and formatted salary.
Sample Output:
Employee Details: ID: 15 Name: Caelius Dathan Salary: $4900.00
Flowchart:
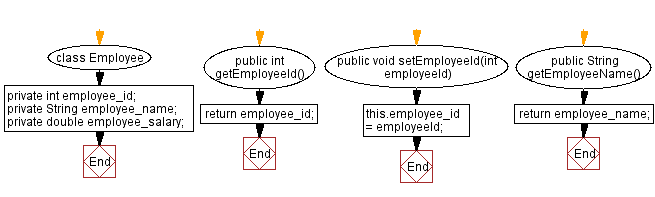
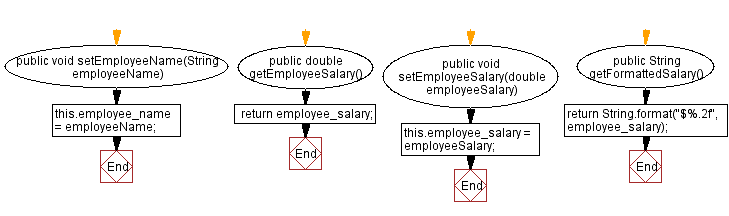
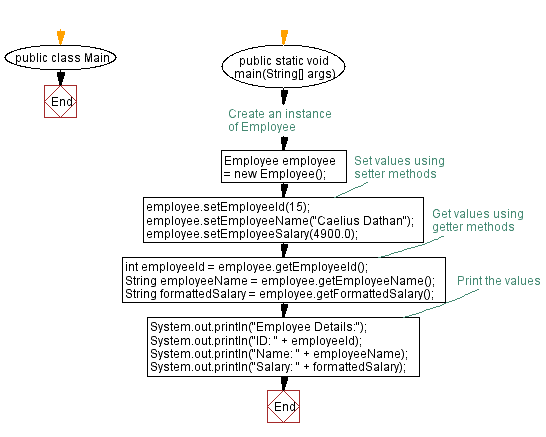
Java Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Implementing a circle class with getter, setter, and calculation methods.
Next: Implementing a circle class with getter, setter, and calculation methods.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics