Java Encapsulation: Implementing Rectangle Class with Getter and Setter Methods
Write a Java program to create a class called Rectangle with private instance variables length and width. Provide public getter and setter methods to access and modify these variables.
Sample Solution:
Java Code:
// Rectangle.java
// Rectangle Class
class Rectangle {
// Declare a private double variable for length
private double length;
// Declare a private double variable for width
private double width;
// Getter method for length
public double getLength() {
return length;
}
// Setter method for length
public void setLength(double length) {
this.length = length;
}
// Getter method for width
public double getWidth() {
return width;
}
// Setter method for width
public void setWidth(double width) {
this.width = width;
}
}
// Main.java
// Main Class
public class Main {
public static void main(String[] args) {
// Create an instance of Rectangle
Rectangle rectangle = new Rectangle();
// Set values using setter methods
rectangle.setLength(6.7);
rectangle.setWidth(12.0);
// Get values using getter methods
double length = rectangle.getLength();
double width = rectangle.getWidth();
// Print the values
System.out.println("Length: " + length);
System.out.println("Width: " + width);
}
}
Output:
Length: 6.7 Width: 12.0
Explanation:
In the above exercise,
the Rectangle class encapsulates the private instance variables length and width. The getLength() and getWidth() methods are public getter methods that allow other classes to access length and width values. The setLength() and setWidth() methods are public setter methods that allow other classes to modify length and width values.
In the Main class, an object rectangle of the Rectangle class is created. The setter methods (setLength() and setWidth()) are used to set length and width values. The getter methods (getLength() and getWidth()) are used to retrieve length and width values.
We then use System.out.println() statements to print the length and width values.
Flowchart:
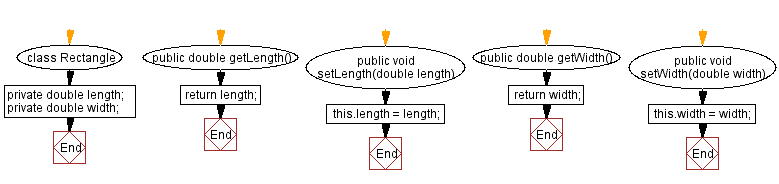
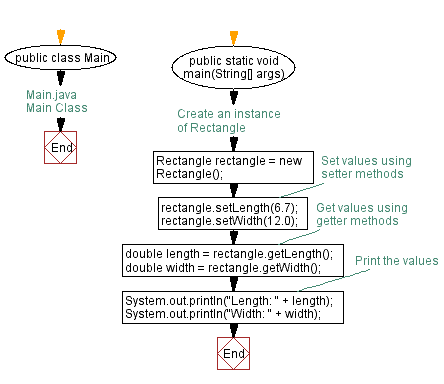
For more Practice: Solve these Related Problems:
- Write a Java program where the "Rectangle" class prevents setting a negative length or width.
- Write a Java program where the "Rectangle" class includes a method to check if it is a square.
- Write a Java program where the "Rectangle" class calculates the diagonal length.
- Write a Java program where the "Rectangle" class supports dynamic resizing while keeping the aspect ratio constant.
Java Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Implementing the BankAccount Class with Getter and Setter Methods.
Next: Implementing an Employee Class with Getter and Setter Methods.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.