Java: Get the information of a given time
Write a Java program to get information about a given time.
Sample Solution:
Java Code:
import java.time.*;
public class DateParseFormatExercise27 {
public static void main(String[] args) {
LocalTime time = LocalTime.of(12, 24, 32);
int hour = time.getHour();
int minute = time.getMinute();
int second = time.getSecond();
System.out.println("\nCurrent Local time: " + time);
System.out.println("Hour: " + hour);
System.out.println("Minute: " + minute);
System.out.println("Second: " +second+"\n");
}
}
Sample Output:
Current Local time: 12:24:32 Hour: 12 Minute: 24 Second: 32
Pictorial Presentation:
Flowchart:
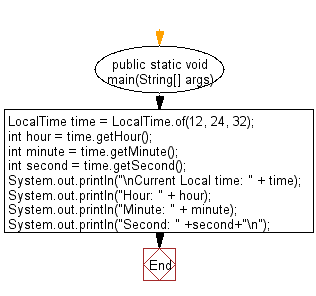
For more Practice: Solve these Related Problems:
- Write a Java program to extract and display the hour, minute, second, and nanosecond from a given time.
- Write a Java program to parse a time string and output detailed time components.
- Write a Java program to retrieve time information from a LocalTime object and format it for display.
- Write a Java program to display detailed information of a user-specified time, including the AM/PM indicator.
Java Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a Java program to to get the information of current/given month.
Next: Write a Java program to display the date time information before some hours and minutes from current date time.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.