Java: Get the information of current or given month
Write a Java program to get the information of the current/given month.
Sample format :
Integer value of the current month: 2 Length of the month: 28 Maximum length of the month: 29 First month of the Quarter: JANUARY
Sample Solution:
Java Code:
import java.time.*;
public class DateParseFormatExercise26 {
public static void main(String[] args) {
// information about the month
LocalDate ldt = LocalDate.of(2016, Month.FEBRUARY, 10);
Month mn = ldt.getMonth(); // FEBRUARY
int mnIntValue = mn.getValue(); // 2
int minLength = mn.minLength(); // 28
int maxLength = mn.maxLength(); // 29
Month firstMonthOfQuarter = mn.firstMonthOfQuarter(); // JANUARY
System.out.println("\nInteger value of the current month: " + mnIntValue);
System.out.println("Length of the month: " + minLength);
System.out.println("Maximum length of the month: " + maxLength);
System.out.println("First month of the Quarter: " + firstMonthOfQuarter+"\n");
}
}
Sample Output:
Integer value of the current month: 2 Length of the month: 28 Maximum length of the month: 29 First month of the Quarter: JANUARY
Pictorial Presentation:
Flowchart:
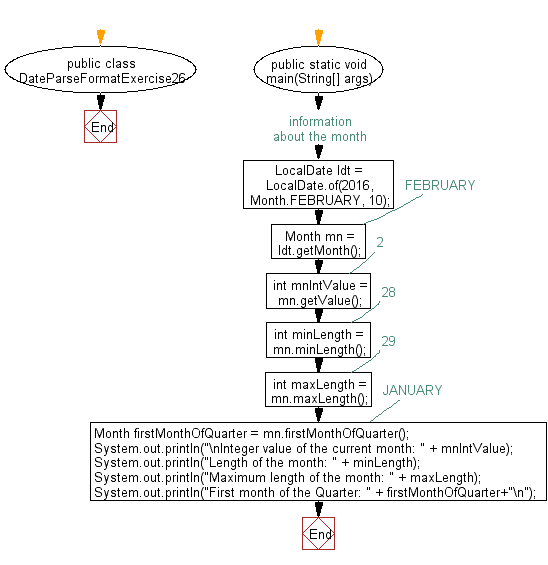
Java Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a Java program to get the information of current/given year.
Next: Write a Java program to get the information of a given time.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics