Java: Check a year is a leap year or not
Java DateTime, Calendar: Exercise-18 with Solution
Write a Java program to check if a year is a leap year or not.
Sample Solution:
Java Code:
public class Exercise18 {
public static void main(String[] args)
{
//year to leap year or not
int year = 2016;
System.out.println();
if((year % 400 == 0) || ((year % 4 == 0) && (year % 100 != 0)))
System.out.println("Year " + year + " is a leap year");
else
System.out.println("Year " + year + " is not a leap year");
System.out.println();
}
}
Sample Output:
Year 2016 is a leap year
Pictorial Presentation:
Flowchart:
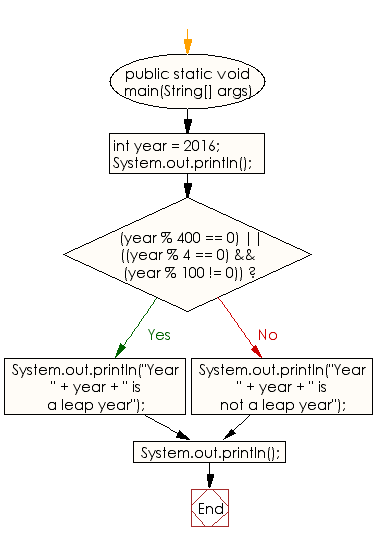
Sample Solution:
Alternate Code :
import java.time.*;
import java.util.*;
public class Exercise18 {
public static void main(String[] args)
{
LocalDate today = LocalDate.now();
if(today.isLeapYear())
{
System.out.println("This year is Leap year");
}
else
{
System.out.println("This year is not a Leap year");
}
}
}
Flowchart:
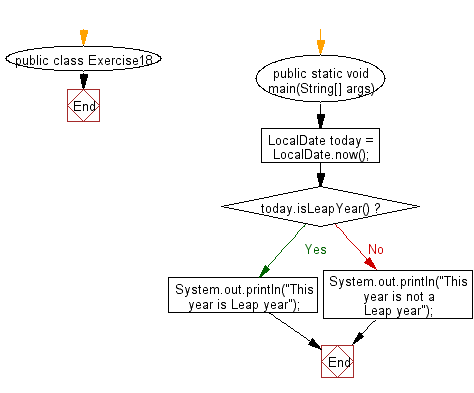
Java Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a Java program to get a date before and after 1 year compares to the current date.
Next: Write a Java program to get year and months between two dates.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics