Java: Reads a number and display the square, cube, and fourth power
Compute Square, Cube, and Fourth Power
Write a Java program that reads a number and displays the square, cube, and fourth power.
Test Data
Input the side length value: 15
Sample Solution:
Java Code:
import java.util.Scanner;
public class Exercise8 {
public static void main(String[] args)
{
Scanner in = new Scanner(System.in);
System.out.print("Input the side length value: ");
double val = in.nextDouble();
System.out.printf("Square: %12.2f\n", val * val);
System.out.printf("Cube: %14.2f\n", val * val * val);
System.out.printf("Fourth power: %6.2f\n", Math.pow(val, 4));
}
}
Sample Output:
Input the side length value: 15 Square: .2f Cube: .2f Fourth power: 50625.00
Flowchart:
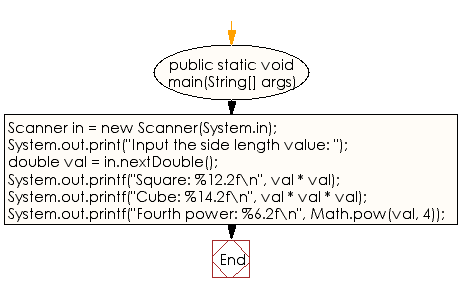
For more Practice: Solve these Related Problems:
- Write a Java program to compute the square, cube, and fourth power of a number using recursive methods.
- Write a Java program to compute and display the square, cube, and fourth power of numbers in a given range using parallel streams.
- Write a Java program to compute the powers of a number without using the Math.pow() method by implementing your own exponentiation function.
- Write a Java program to compute square, cube, and fourth power and then compare the results with those obtained using bitwise operations where applicable.
Go to:
PREV : Calculate Speeds (m/s, km/h, mph).
NEXT : Arithmetic Operations on Two Integers.
JavaCode Editor:
Improve this sample solution and post your code through Disqus
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.