Java: Compute body mass index (BMI)
Compute BMI
Write a Java program to compute the body mass index (BMI).
BMI: The BMI is defined as the body mass divided by the square of the body height, and is universally expressed in units of kg/m2, resulting from mass in kilograms and height in metres.
Test Data
Input weight in pounds: 452
Input height in inches: 72
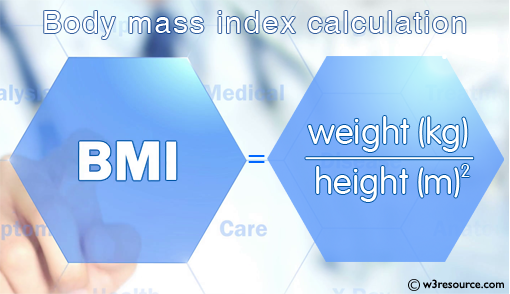
Sample Solution:
Java Code:
import java.util.Scanner;
public class Exercise6 {
public static void main(String[] Strings) {
Scanner input = new Scanner(System.in);
System.out.print("Input weight in pounds: ");
double weight = input.nextDouble();
System.out.print("Input height in inches: ");
double inches = input.nextDouble();
double BMI = weight * 0.45359237 / (inches * 0.0254 * inches * 0.0254);
System.out.print("Body Mass Index is " + BMI+"\n");
}
}
Sample Output:
Input weight in pounds: 452 Input height in inches: 72 Body Mass Index is 61.30159143458721
Flowchart:
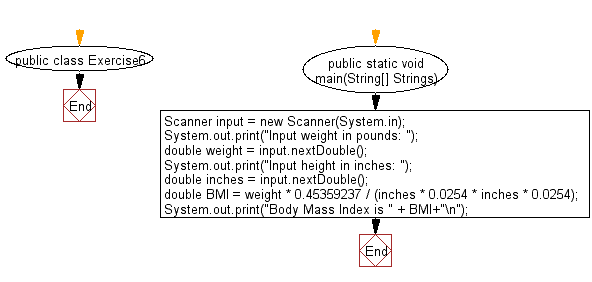
For more Practice: Solve these Related Problems:
- Write a Java program to compute BMI using both metric and imperial units, allowing the user to choose the input format.
- Write a Java program to validate weight and height inputs for BMI calculation and handle exceptions for invalid entries.
- Write a Java program to compute BMI and then classify the result into underweight, normal, overweight, or obese categories.
- Write a Java program to compute BMI for a list of individuals read from a file and output a summary report with statistical analysis.
Java Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a Java program that prints the current time in GMT.
Next: Write a Java program to display the speed, in meters per second, kilometers per hour and miles per hour.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.