Java: Find Vowel or Consonant
Java Conditional Statement: Exercise-8 with Solution
Write a Java program that requires the user to enter a single character from the alphabet. Print Vowel or Consonant, depending on user input. If the user input is not a letter (between a and z or A and Z), or is a string of length > 1, print an error message.
Test Data
Input an alphabet: p
Pictorial Presentation:
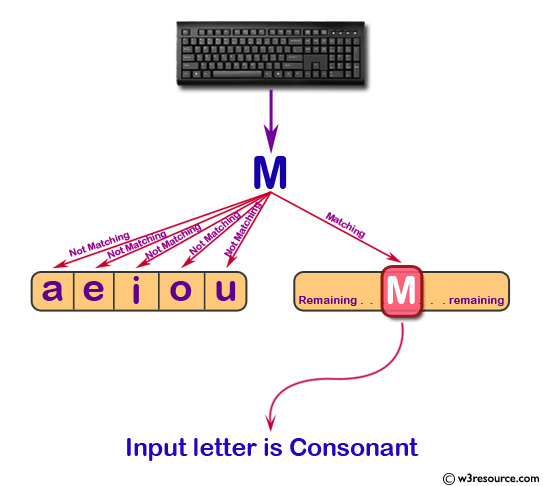
Sample Solution:
Java Code:
import java.util.Scanner;
public class Exercise8 {
public static void main(String[] args)
{
Scanner in = new Scanner(System.in);
System.out.print("Input an alphabet: ");
String input = in.next().toLowerCase();
boolean uppercase = input.charAt(0) >= 65 && input.charAt(0) <= 90;
boolean lowercase = input.charAt(0) >= 97 && input.charAt(0) <= 122;
boolean vowels = input.equals("a") || input.equals("e") || input.equals("i")
|| input.equals("o") || input.equals("u");
if (input.length() > 1)
{
System.out.println("Error. Not a single character.");
}
else if (!(uppercase || lowercase))
{
System.out.println("Error. Not a letter. Enter uppercase or lowercase letter.");
}
else if (vowels)
{
System.out.println("Input letter is Vowel");
}
else
{
System.out.println("Input letter is Consonant");
}
}
}
Sample Output:
Input an alphabet: P Input letter is Consonant
Flowchart:
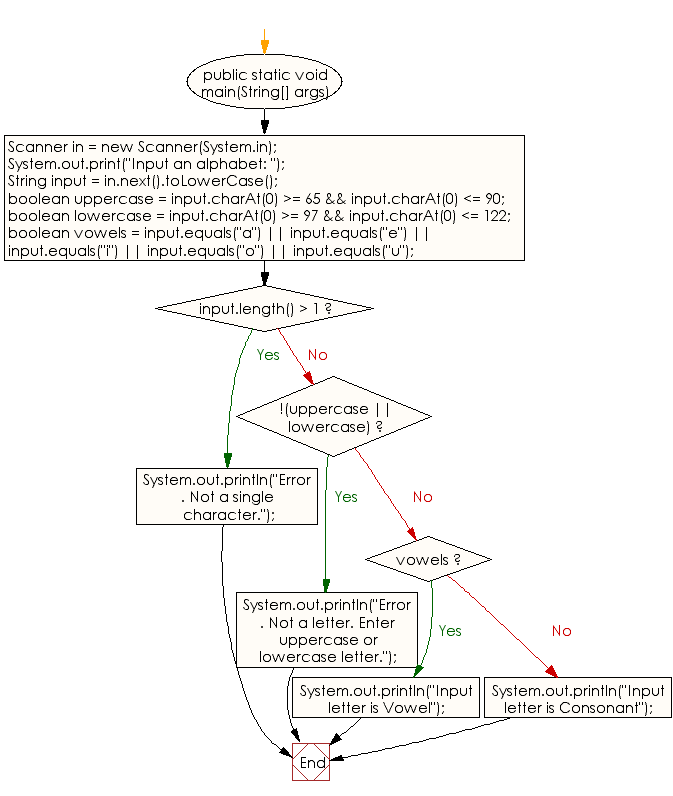
Java Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Java program to find the number of days in a month.
Next: Write a Java program that takes a year from user and print whether that year is a leap year or not.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/java-exercises/conditional-statement/java-conditional-statement-exercise-8.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics