Java: Test two floating-point numbers are same upto three decimal places
Compare Floats Up to Three Decimals
Write a Java program that reads two floating-point numbers and tests whether they are the same up to three decimal places.
Test Data
Input floating-point number: 25.586
Input floating-point another number: 25.589
Sample Solution:
Java Code:
import java.util.Scanner;
public class Exercise6 {
public static void main(String[] args)
{
Scanner in = new Scanner(System.in);
System.out.print("Input floating-point number: ");
double x = in.nextDouble();
System.out.print("Input floating-point another number: ");
double y = in.nextDouble();
x = Math.round(x * 1000);
x = x / 1000;
y = Math.round(y * 1000);
y = y / 1000;
if (x == y)
{
System.out.println("They are the same up to three decimal places");
}
else
{
System.out.println("They are different");
}
}
}
Sample Output:
Input floating-point number: 25.586 Input floating-point another number: 25.589 They are different
Flowchart:
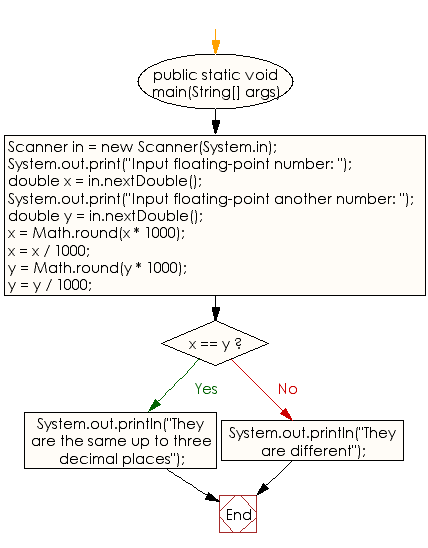
Java Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Java program that keeps a number from the user and generates an integer between 1 and 7 and displays the name of the weekday.
Next: Write a Java program to find the number of days in a month.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics