Java: Accepts two floatingpoint numbers and checks whether they are the same up to two decimal places
Java Conditional Statement: Exercise-32 with Solution
Write a Java program that accepts two floatingpoint numbers and checks whether they are the same up to two decimal places.
Test Data
Input first floatingpoint number: 1235
Input second floatingpoint number: 2534
Pictorial Presentation:
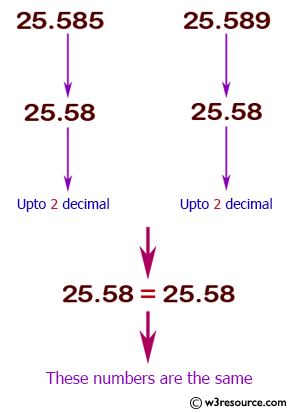
Sample Solution:
Java Code:
import java.util.Scanner;
public class Exercise32 {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
System.out.print("Input first floatingpoint number: ");
double num1 = input.nextDouble();
System.out.print("Input second floatingpoint number: ");
double num2 = input.nextDouble();
input.close();
if (Math.abs(num1 - num2) <= 0.01) {
System.out.println("These numbers are the same.");
}
else {
System.out.println("These numbers are different.");
}
}
}
Sample Output:
Input first floatingpoint number: 1235 Input second floatingpoint number: 2534 These numbers are different.
Flowchart:
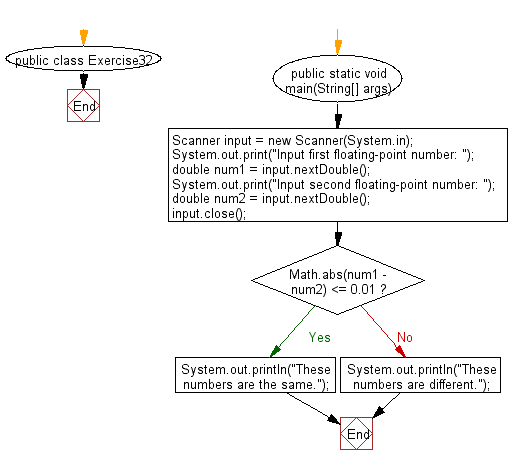
Java Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program that accepts three numbers from the user and prints "increasing" if the numbers are in increasing order, "decreasing" if the numbers are in decreasing order, and "Neither increasing or decreasing order" otherwise.
Next: Java Array exercises
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics