Java: Compare two tree sets
8. Compare TreeSets
Write a Java program to compare two tree sets.
Sample Solution:
Java Code:
import java.util.TreeSet;
import java.util.Iterator;
public class Exercise8 {
public static void main(String[] args) {
// Create a empty tree set
TreeSet<String> t_set1 = new TreeSet<String>();
// use add() method to add values in the tree set
t_set1.add("Red");
t_set1.add("Green");
t_set1.add("Black");
t_set1.add("White");
System.out.println("Free Tree set: "+t_set1);
TreeSet<String> t_set2 = new TreeSet<String>();
t_set2.add("Red");
t_set2.add("Pink");
t_set2.add("Black");
t_set2.add("Orange");
System.out.println("Second Tree set: "+t_set2);
//comparison output in tree set
TreeSet<String> result_set = new TreeSet<String>();
for (String element : t_set1){
System.out.println(t_set2.contains(element) ? "Yes" : "No");
}
}
}
Sample Output:
Free Tree set: [Black, Green, Red, White] Second Tree set: [Black, Orange, Pink, Red] Yes No Yes No
Flowchart:
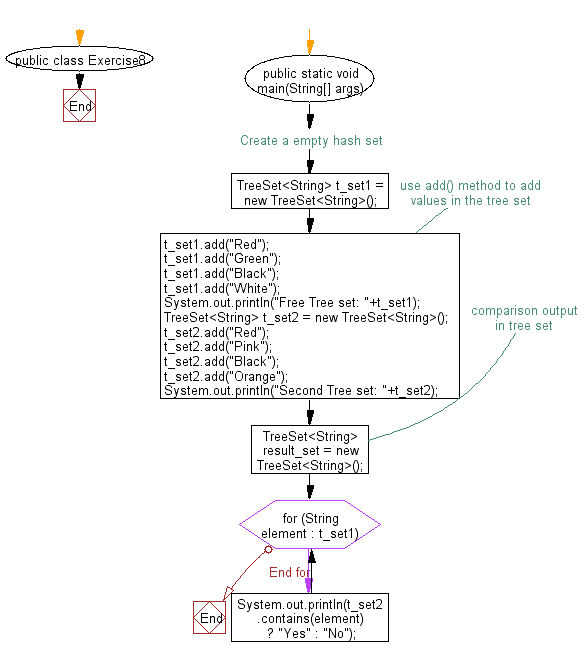
For more Practice: Solve these Related Problems:
- Write a Java program to compare two TreeSets for equality by checking if they contain the same elements in the same order.
- Write a Java program to compute the union and intersection of two TreeSets and then compare the sizes of the results.
- Write a Java program to use Java streams to compare two TreeSets and return a boolean indicating whether they are identical.
- Write a Java program to compare two TreeSets of custom objects using a custom comparator for equality.
Go to:
PREV : TreeSet Size.
NEXT : Elements Less Than 7 in TreeSet.
Java Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.