Java: Clone a tree set list to another tree set
6. Clone TreeSet
Write a Java program to clone a tree set list to another tree set.
Sample Solution:
Java Code:
import java.util.TreeSet;
import java.util.Iterator;
public class Exercise6 {
public static void main(String[] args) {
// create an empty tree set
TreeSet<String> t_set = new TreeSet<String>();
// use add() method to add values in the tree set
t_set.add("Red");
t_set.add("Green");
t_set.add("Black");
t_set.add("Pink");
t_set.add("orange");
System.out.println("Original tree set:" + t_set);
TreeSet<String> new_t_set = (TreeSet<String>)t_set.clone();
System.out.println("Cloned tree list: " + t_set);
}
}
Sample Output:
Note: Exercise6.java uses unchecked or unsafe operations. Note: Recompile with -Xlint:unchecked for details. Original tree set:[Black, Green, Pink, Red, orange] Cloned tree list: [Black, Green, Pink, Red, orange]
Flowchart:
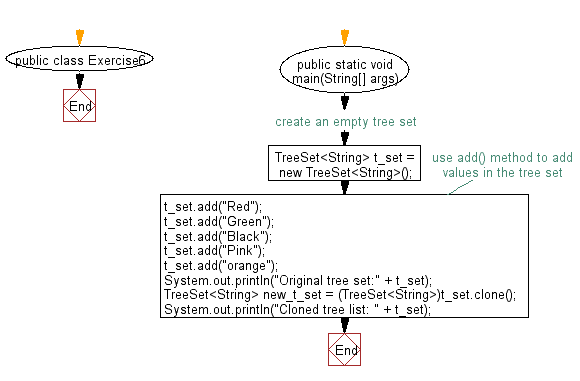
For more Practice: Solve these Related Problems:
- Write a Java program to clone a TreeSet using the clone() method and verify that modifications in the clone do not affect the original.
- Write a Java program to perform a deep copy of a TreeSet of custom objects by implementing the Cloneable interface.
- Write a Java program to clone a TreeSet using Java streams and then compare the cloned set with the original for equality.
- Write a Java program to clone a TreeSet and then sort the clone in reverse order using a custom comparator.
Go to:
PREV : Get First and Last TreeSet Elements.
NEXT : TreeSet Size.
Java Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.