Java: Insert elements into the linked list at the first and last position
Write a Java program to insert elements into the linked list at the first and last positions.
Sample Solution:-
Java Code:
import java.util.LinkedList;
public class Exercise6 {
public static void main(String[] args) {
// create an empty linked list
LinkedList<String> l_list = new LinkedList<String>();
// use add() method to add values in the linked list
l_list.add("Red");
l_list.add("Green");
l_list.add("Black");
System.out.println("Original linked list:" + l_list);
// Add an element at the beginning
l_list.addFirst("White");
// Add an element at the end of list
l_list.addLast("Pink");
System.out.println("Final linked list:" + l_list);
}
}
Sample Output:
Original linked list:[Red, Green, Black] Final linked list:[White, Red, Green, Black, Pink]
Pictorial Presentation:
Flowchart:
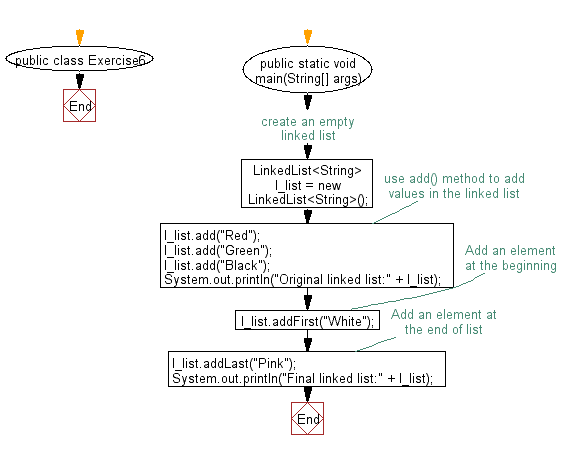
For more Practice: Solve these Related Problems:
- Write a Java program to insert a list of elements at both the beginning and end of a linked list and then merge them.
- Write a Java program to implement methods that separately insert an element at the head and tail, then verify the list order.
- Write a Java program to insert elements at the first and last positions and then reverse the list to check correctness.
- Write a Java program to use recursion to insert elements at both ends of a linked list and then print the resulting list.
Java Code Editor:
Contribute your code and comments through Disqus.
Previous: Insert the specified element at the specified position in the linked list.
Next: Insert the specified element at the front of a linked list.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.