Java: Check if a particular element exists in a linked list
Write a Java program to check if a particular element exists in a linked list.
Sample Solution:-
Java Code:
import java.util.*;
public class Exercise22 {
public static void main(String[] args) {
// create an empty linked list
LinkedList <String> c1 = new LinkedList <String> ();
c1.add("Red");
c1.add("Green");
c1.add("Black");
c1.add("White");
c1.add("Pink");
System.out.println("Original linked list: " + c1);
// Checks whether the color "Green" exists or not.
if (c1.contains("Green")) {
System.out.println("Color Green is present in the linked list.");
} else {
System.out.println("Color Green is not present in the linked list.");
}
// Checks whether the color "Orange" exists or not.
if (c1.contains("Orange")) {
System.out.println("Color Orange is present in the linked list.");
} else {
System.out.println("Color Orange is not present in the linked list.");
}
}
}
Sample Output:
Original linked list: [Red, Green, Black, White, Pink] Color Green is present in the linked list. Color Orange is not present in the linked list.
Pictorial Presentation:
Flowchart:
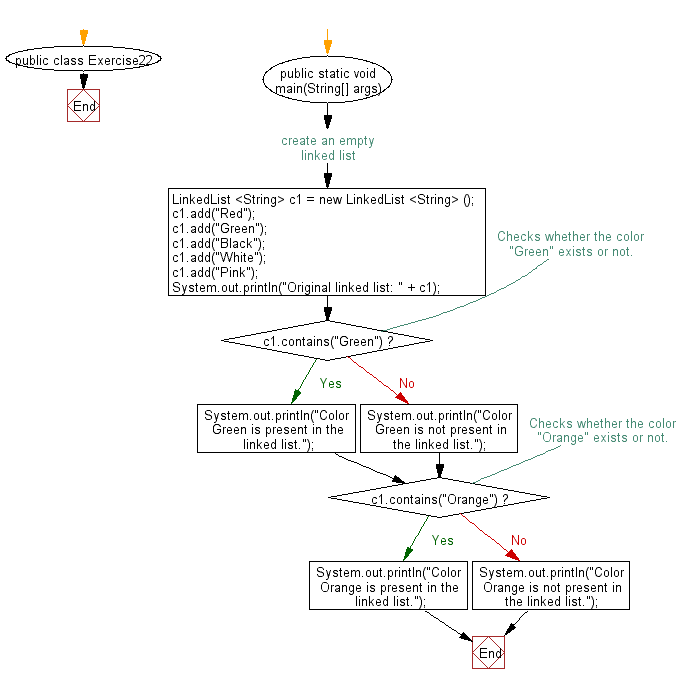
Java Code Editor:
Contribute your code and comments through Disqus.
Previous: Retrieve but does not remove, the last element of a linked list.
Next: Convert a linked list to array list.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics