Java: Retrieve but does not remove, the first element of a linked list
Write a Java program to retrieve, but not remove, the first element of a linked list.
Sample Solution:-
Java Code:
import java.util.*;
public class Exercise20 {
public static void main(String[] args) {
// create an empty linked list
LinkedList <String> c1 = new LinkedList <String> ();
c1.add("Red");
c1.add("Green");
c1.add("Black");
c1.add("White");
c1.add("Pink");
System.out.println("Original linked list: " + c1);
// Retrieve but does not remove, the first element of a linked list
String x = c1.peekFirst();
System.out.println("First element in the list: " + x);
System.out.println("Original linked list: " + c1);
}
}
Sample Output:
Original linked list: [Red, Green, Black, White, Pink] First element in the list: Red Original linked list: [Red, Green, Black, White, Pink]
Pictorial Presentation:
Flowchart:
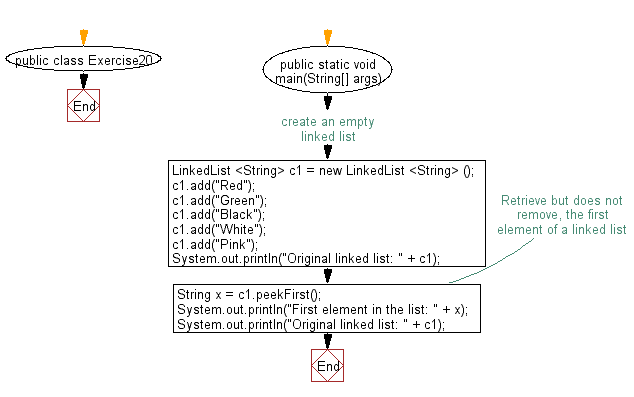
For more Practice: Solve these Related Problems:
- Write a Java program to retrieve the first element of a linked list using peek() without removing it.
- Write a Java program to implement a method that returns the first element without modifying the linked list structure.
- Write a Java program to use recursion to access the head element of a linked list and print it.
- Write a Java program to compare the first element retrieved with the element obtained after a simulated pop and push.
Java Code Editor:
Contribute your code and comments through Disqus.
Previous: Remove and return the first element of a linked list.
Next: Retrieve but does not remove, the last element of a linked list.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.