Java: Convert a hash set to an array
7. Convert HashSet to Array
Write a Java program to convert a hash set to an array.
Sample Solution:
Java Code:
import java.util.*;
public class Exercise7 {
public static void main(String[] args) {
// Create a empty hash set
HashSet<String> h_set = new HashSet<String>();
// use add() method to add values in the hash set
h_set.add("Red");
h_set.add("Green");
h_set.add("Black");
h_set.add("White");
h_set.add("Pink");
h_set.add("Yellow");
System.out.println("Original Hash Set: " + h_set);
// Creating an Array
String[] new_array = new String[h_set.size()];
h_set.toArray(new_array);
// Displaying Array elements
System.out.println("Array elements: ");
for(String element : new_array){
System.out.println(element);
}
}
}
Sample Output:
Original Hash Set: [Red, White, Pink, Yellow, Black, Green] Array elements: Red White Pink Yellow Black Green
Pictorial Presentation:
Flowchart:
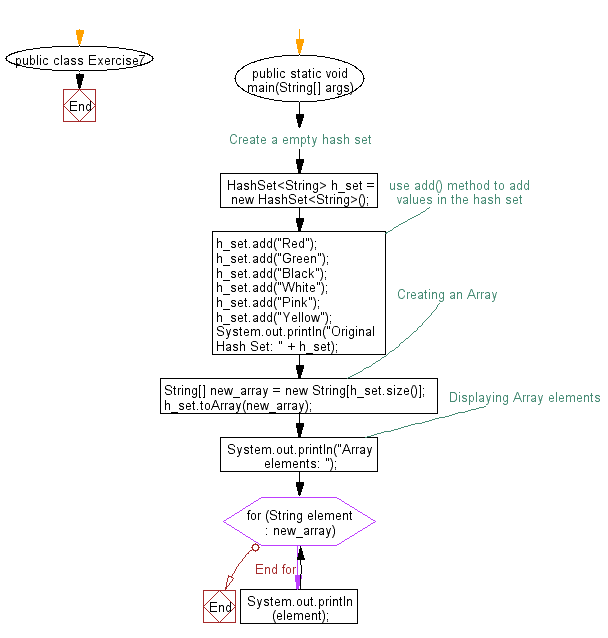
For more Practice: Solve these Related Problems:
- Write a Java program to convert a HashSet of integers to an array and then sort the array.
- Write a Java program to convert a HashSet to an array using the toArray() method and then search for a specific element in the array.
- Write a Java program to convert a HashSet to an array and then iterate over it using a for-each loop.
- Write a Java program to convert a HashSet to an array, then compute and print the sum of its elements.
Go to:
PREV : Clone HashSet.
NEXT : Convert HashSet to TreeSet.
Java Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.