Java: Get the number of elements in a hash set
Java Collection, HashSet Exercises: Exercise-3 with Solution
Write a Java program to get the number of elements in a hash set.
Sample Solution:
Java Code:
import java.util.*;
import java.util.Iterator;
public class Exercise3 {
public static void main(String[] args) {
// Create a empty hash set
HashSet<String> h_set = new HashSet<String>();
// use add() method to add values in the hash set
h_set.add("Red");
h_set.add("Green");
h_set.add("Black");
h_set.add("White");
h_set.add("Pink");
h_set.add("Yellow");
System.out.println("Original Hash Set: " + h_set);
System.out.println("Size of the Hash Set: " + h_set.size());
}
}
Sample Output:
Original Hash Set: [Red, White, Pink, Yellow, Black, Green] Size of the Hash Set: 6
Pictorial Presentation:
Flowchart:
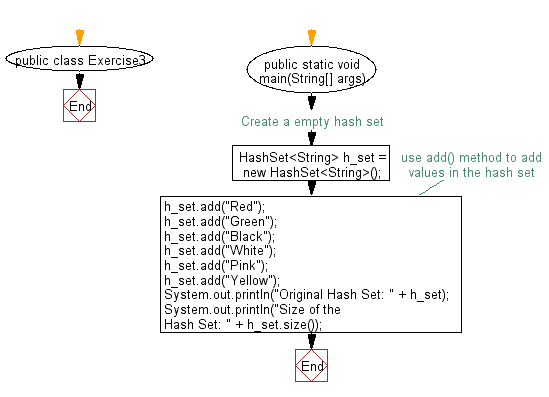
Java Code Editor:
Contribute your code and comments through Disqus.
Previous: Iterate through all elements in a hash list.
Next: Empty an hash set.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/java-exercises/collection/java-collection-hash-set-exercise-3.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics