Java: Iterate through all elements in a hash list
Java Collection, HashSet Exercises: Exercise-2 with Solution
Write a Java program to iterate through all elements in a hash list.
Sample Solution:
Java Code:
import java.util.*;
import java.util.Iterator;
public class Exercise2 {
public static void main(String[] args) {
// Create a empty hash set
HashSet<String> h_set = new HashSet<String>();
// use add() method to add values in the hash set
h_set.add("Red");
h_set.add("Green");
h_set.add("Black");
h_set.add("White");
h_set.add("Pink");
h_set.add("Yellow");
// set Iterator
Iterator<String> p = h_set.iterator();
// Iterate the hash set
while (p.hasNext()) {
System.out.println(p.next());
}
}
}
Sample Output:
Red White Pink Yellow Black Green
Flowchart:
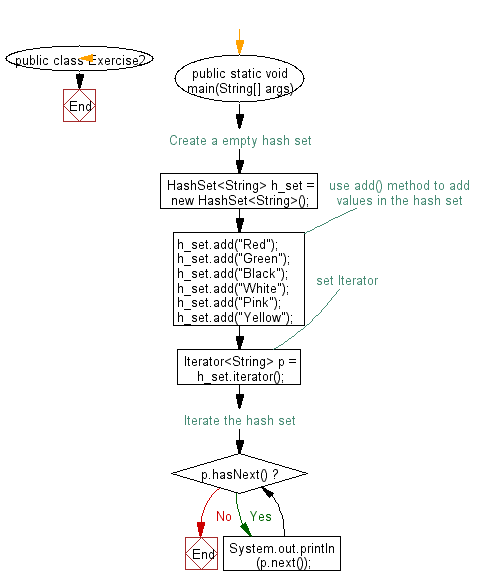
Java Code Editor:
Contribute your code and comments through Disqus.
Previous: Append the specified element to the end of a hash set.
Next: Get the number of elements in a hash set.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/java-exercises/collection/java-collection-hash-set-exercise-2.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics