Java: Remove all of the elements from a hash set
Java Collection, HashSet Exercises: Exercise-12 with Solution
Write a Java program to remove all elements from a hash set.
Sample Solution:
Java Code:
import java.util.*;
public class Exercise12 {
public static void main(String[] args) {
// Create a empty hash set
HashSet<String> h_set = new HashSet<String>();
// use add() method to add values in the hash set
h_set.add("Red");
h_set.add("Green");
h_set.add("Black");
h_set.add("White");
System.out.println("Original hash set contains: "+ h_set);
// clear() method removes all the elements from a hash set
// and the set becomes empty.
h_set.clear();
// Display original hash set content again
System.out.println("HashSet content: "+h_set);
}
}
Sample Output:
Original hash set contains: [Red, White, Black, Green] HashSet content: []
Pictorial Presentation:
Flowchart:
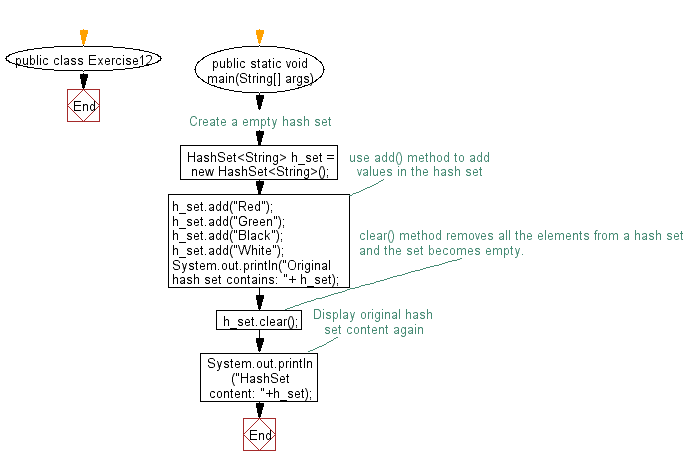
Java Code Editor:
Contribute your code and comments through Disqus.
Previous: Compare two sets and retain elements which are same on both sets.
Next: Create a new tree set, add some colors and print out the tree set.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/java-exercises/collection/java-collection-hash-set-exercise-12.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics