Java: Copy one array list into another
Java Collection, ArrayList Exercises: Exercise-9 with Solution
Write a Java program to copy one array list into another.
Sample Solution:-
Java Code:
import java.util.*;
public class Exercise9 {
public static void main(String[] args) {
List<String> List1 = new ArrayList<String>();
List1.add("1");
List1.add("2");
List1.add("3");
List1.add("4");
System.out.println("List1: " + List1);
List<String> List2 = new ArrayList<String>();
List2.add("A");
List2.add("B");
List2.add("C");
List2.add("D");
System.out.println("List2: " + List2);
// Copy List2 to List1
Collections.copy(List1, List2);
System.out.println("Copy List to List2,\nAfter copy:");
System.out.println("List1: " + List1);
System.out.println("List2: " + List2);
}
}
Sample Output:
List1: [1, 2, 3, 4] List2: [A, B, C, D] Copy List to List2, After copy: List1: [A, B, C, D] List2: [A, B, C, D]
Flowchart:
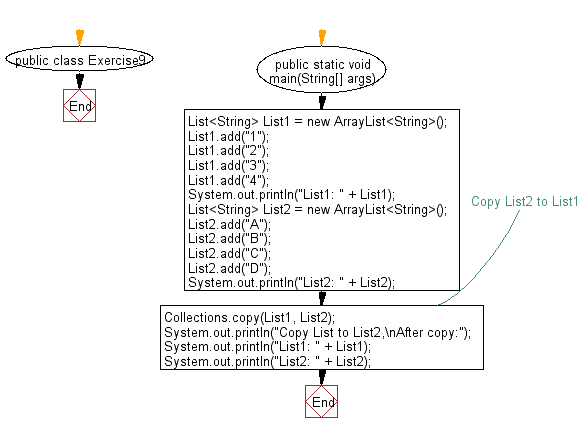
Java Code Editor:
Contribute your code and comments through Disqus.
Previous: Sort a given array list.
Next: Shuffle elements in a array list.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/java-exercises/collection/java-collection-exercise-9.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics