Java: Insert an element into the array list at the first position
Java Collection, ArrayList Exercises: Exercise-3 with Solution
Write a Java program to insert an element into the array list at the first position.
Pictorial Presentation:
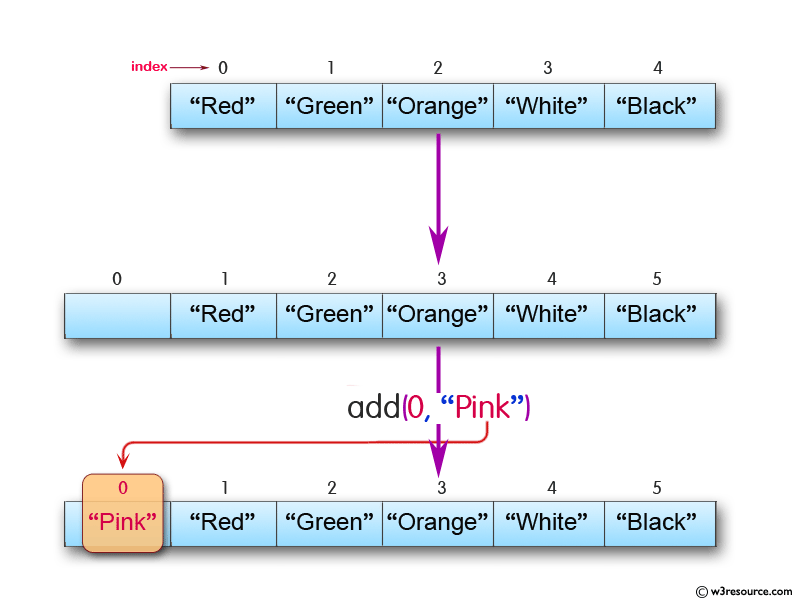
Sample Solution:-
Java Code:
import java.util.*;
public class Exercise3 {
public static void main(String[] args) {
// Creae a list and add some colors to the list
List<String> list_Strings = new ArrayList<String>();
list_Strings.add("Red");
list_Strings.add("Green");
list_Strings.add("Orange");
list_Strings.add("White");
list_Strings.add("Black");
// Print the list
System.out.println(list_Strings);
// Now insert a color at the first and last position of the list
list_Strings.add(0, "Pink");
list_Strings.add(5, "Yellow");
// Print the list
System.out.println(list_Strings);
}
}
Sample Output:
Note: Exercise3.java uses unchecked or unsafe operations. Note: Recompile with -Xlint:unchecked for details. [Red, Green, Orange, White, Black] [Pink, Red, Green, Orange, White, Yellow, Black]
Flowchart:
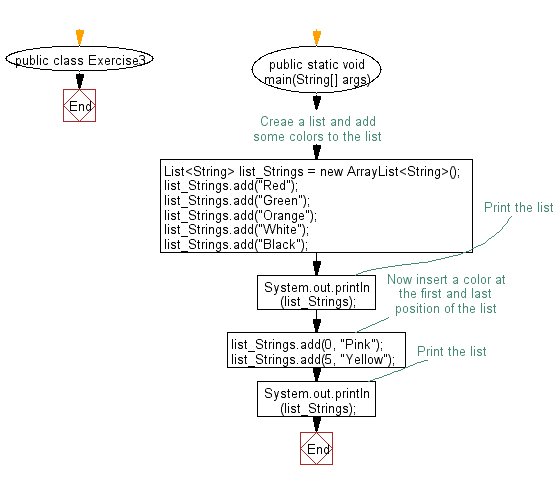
Java Code Editor:
Contribute your code and comments through Disqus.
Previous: Iterate through all elements in a array list.
Next: Retrieve an element from a given array list.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/java-exercises/collection/java-collection-exercise-3.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics