Java: Empty an array list
17. Clear ArrayList
Write a Java program to empty an array list.
Pictorial Presentation:
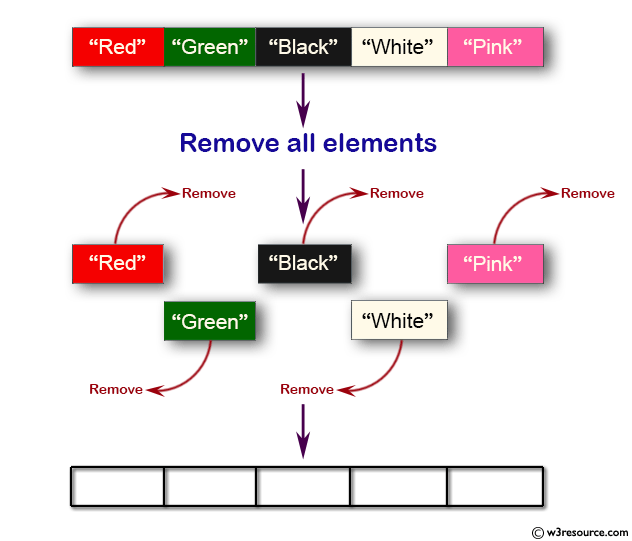
Sample Solution:-
Java Code:
import java.util.ArrayList;
import java.util.Collections;
public class Exercise17 {
public static void main(String[] args) {
ArrayList<String> c1= new ArrayList<String>();
c1.add("Red");
c1.add("Green");
c1.add("Black");
c1.add("White");
c1.add("Pink");
System.out.println("Original array list: " + c1);
c1.removeAll(c1);
System.out.println("Array list after remove all elements "+c1);
}
}
Sample Output:
Original array list: [Red, Green, Black, White, Pink] Array list after remove all elements []
Flowchart:
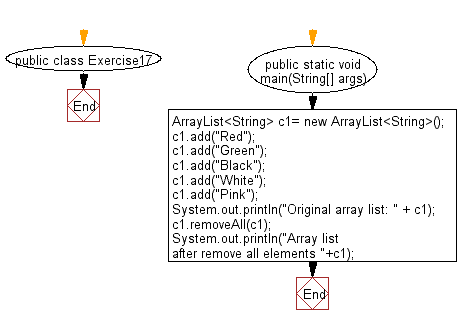
For more Practice: Solve these Related Problems:
- Write a Java program to clear an ArrayList using the clear() method and then check its size.
- Write a Java program to remove all elements from an ArrayList by repeatedly calling remove() in a loop.
- Write a Java program to create a method that empties an ArrayList and returns a boolean indicating success.
- Write a Java program to implement a lambda expression that empties an ArrayList and then verifies if it is empty.
Go to:
PREV : Clone ArrayList.
NEXT : Check if ArrayList is Empty.
Java Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.