Java: Swap two elements in an array list
Write a Java program that swaps two elements in an array list.
Pictorial Presentation:
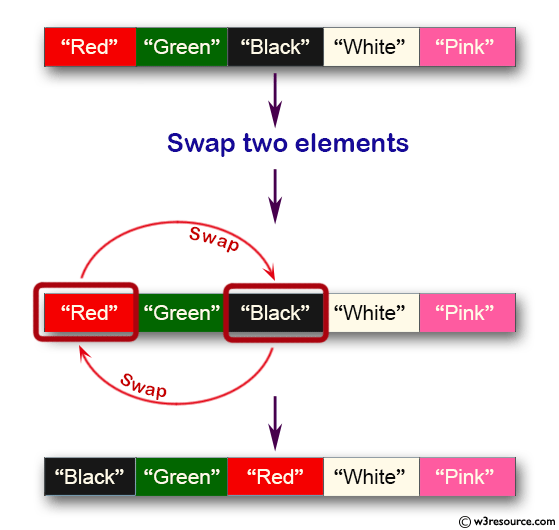
Sample Solution:-
Java Code:
import java.util.ArrayList;
import java.util.Collections;
public class Exercise14 {
public static void main(String[] args) {
ArrayList<String> c1= new ArrayList<String>();
c1.add("Red");
c1.add("Green");
c1.add("Black");
c1.add("White");
c1.add("Pink");
System.out.println("Array list before Swap:");
for(String a: c1){
System.out.println(a);
}
//Swapping 1st(index 0) element with the 3rd(index 2) element
Collections.swap(c1, 0, 2);
System.out.println("Array list after swap:");
for(String b: c1){
System.out.println(b);
}
}
}
Sample Output:
Array list before Swap: Red Green Black White Pink Array list after swap: Black Green Red White Pink
Flowchart:
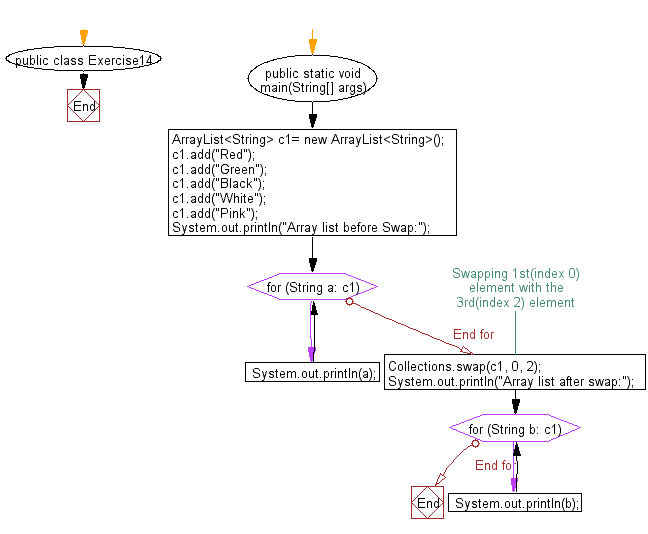
Java Code Editor:
Contribute your code and comments through Disqus.
Previous: Compare two array lists.
Next: Join two array lists.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics