Java: Measure how long some code takes to execute in nanoseconds
Code Execution Time in Nanoseconds
Write a Java program to measure how long code executes in nanoseconds.
Pictorial Presentation:
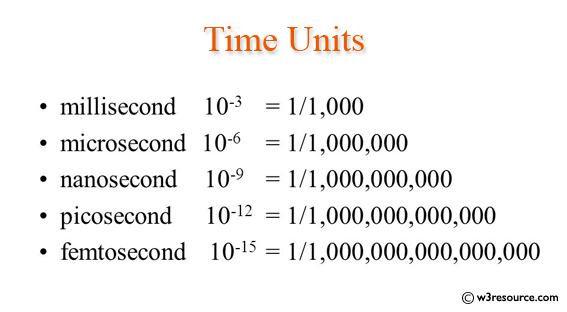
Sample Solution:
Java Code:
import java.lang.*;
public class Exercise91 {
public static void main(String[] args) {
long startTime = System.nanoTime();
// Sample program: Display the first 10 natural numbers.
int i;
System.out.println("The first 10 natural numbers are:\n");
for (i = 1; i <= 10; i++) {
System.out.println(i);
}
// Calculate the elapsed time in nanoseconds.
long estimatedTime = System.nanoTime() - startTime;
System.out.println("Estimated time (in nanoseconds) to get the first 10 natural numbers: " + estimatedTime);
}
}
Sample Output:
The first 10 natural numbers are: 1 2 3 4 5 6 7 8 9 10 Estimated time (in nanoseconds) to get the first 10 natural numbers: 29 1045
Flowchart:
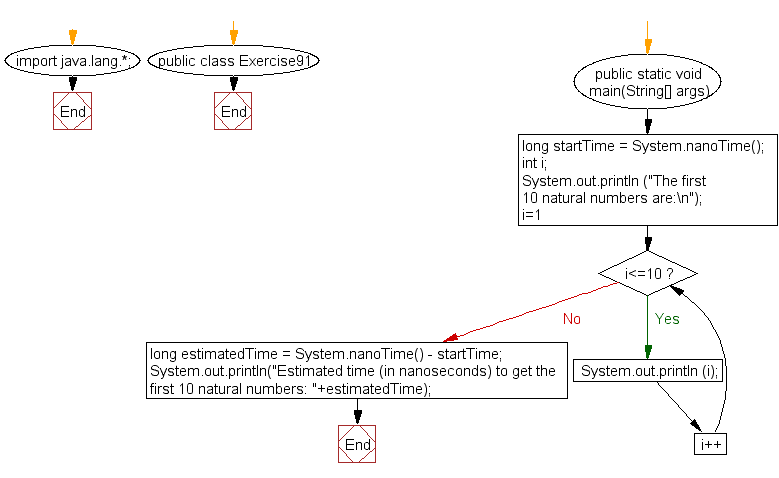
For more Practice: Solve these Related Problems:
- Write a Java program to measure the execution time of sorting an array using different sorting algorithms.
- Write a Java program to compare execution times between iterating a list using a for-loop, an iterator, and a stream.
- Write a Java program to measure the execution time of a recursive Fibonacci function vs an iterative Fibonacci function.
- Write a Java program to measure the execution time of reading a large file using different input methods.
Java Code Editor:
Previous: Write a Java program to get the value of the environment variable PATH, TEMP, USERNAME.
Next: Write a Java program to count the number of even and odd elements in a given array of integers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics