Java: Program than read an integer and calculate the sum of its digits and write the number of each digit of the sum in English
Java Basic: Exercise-87 with Solution
Write a Java program that then reads an integer and calculates the sum of its digits and writes the number of each digit of the sum in English.
Sample Solution:
Java Code:
import java.io.*;
public class Main {
public static void main(String[] args) {
// Create a BufferedReader to read input from the user
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
try {
int sum = 0;
// Read a line of text from the user
String str = br.readLine();
char[] numStr = str.toCharArray();
// Calculate the sum of the digits in the input number
for (int i = 0; i < numStr.length; i++) {
sum += numStr[i] - '0';
}
// Print the original number and call the print_number function
System.out.println("Original Number: " + str);
print_number(sum);
} catch (IOException e) {
e.printStackTrace();
}
}
public static void print_number(int n) {
int x;
int y;
int z;
String[] number = {"zero", "one", "two", "three", "four", "five", "six", "seven", "eight", "nine"};
// Print the sum of the digits of the number
System.out.println("Sum of the digits of the said number: " + n);
if (n < 10) {
// If the number is less than 10, print the corresponding word
System.out.println(number[n]);
} else if (n < 100) {
// If the number is less than 100, split it into tens and ones
x = n / 10;
y = n - x * 10;
System.out.println("In English: " + number[x] + " " + number[y]);
} else {
// If the number is three digits, split it into hundreds, tens, and ones
x = n / 100;
y = (n - x * 100) / 10;
z = n - x * 100 - y * 10;
System.out.println("In English: " + number[x] + " " + number[y] + " " + number[z]);
}
}
}
If input 8
Sample Output:
Original Number: 8 Sum of the digits of the said number: 8 eight
Flowchart:
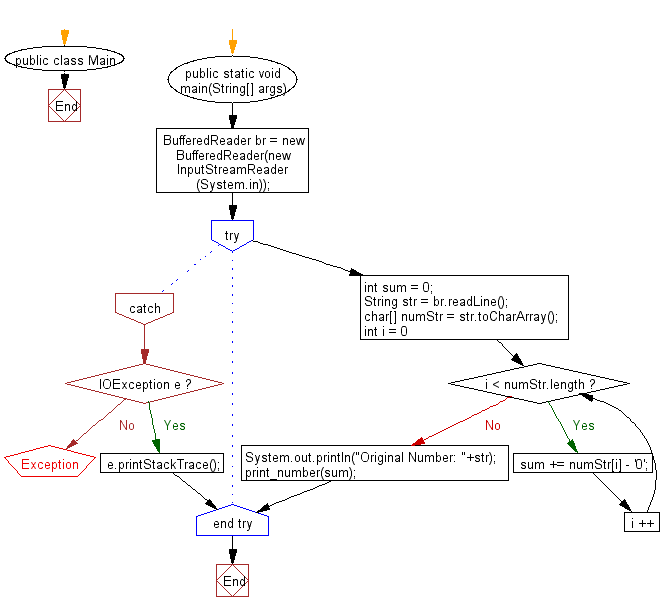
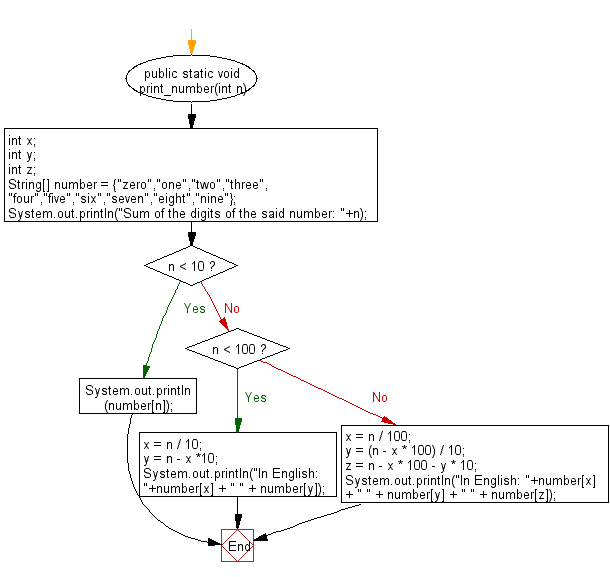
Java Code Editor:
Previous: Write a Java program start with an integer n, divide n by 2 if n is even or multiply by 3 and add 1 if n is odd, repeat the process until n = 1.
Next: Write a Java program to get the current system environment and system properties.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/java-exercises/basic/java-basic-exercise-87.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics