Java: Program to start with an integer n, divide n by 2 if n is even or multiply by 3 and add 1 if n is odd, repeat the process until n = 1
Collatz Conjecture Simulation
Write a Java program starting with an integer n, divide it by 2 if it is even, or multiply it by 3 and add 1 if it is odd. Repeat the process until n = 1.
Sample Solution:
Java Code:
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
// Prompt the user to input the value of n
System.out.println("Input the value of n: ");
// Create a Scanner object to read user input
Scanner in = new Scanner(System.in);
// Read an integer from the user
int n = in.nextInt();
// Continue looping until n becomes 1
while (n != 1) {
// Check if n is even
if (n % 2 == 0) {
n = n / 2; // If even, divide n by 2
} else {
n = (3 * n + 1) / 2; // If odd, perform a calculation
}
}
// Print the final value of n
System.out.println("\nValue of n = " + n);
// Close the Scanner
in.close();
}
}
If input 5
Sample Output:
Input the value of n: 9 Value of n = 1
Flowchart:
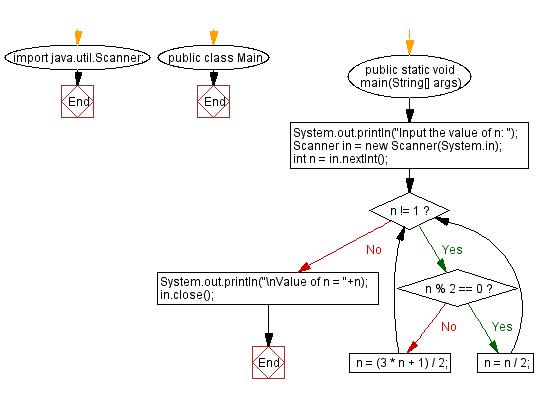
Previous: Write a Java program to check if a string starts with a specified word.
Next: Write a Java program than read an integer and calculate the sum of its digits and write the number of each digit of the sum in English.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics