Java: Multiply corresponding elements of two arrays of integers
Java Basic: Exercise-83 with Solution
Write a Java program to multiply the corresponding elements of two integer arrays.
Sample Solution:
Java Code:
import java.util.*;
public class Exercise83 {
public static void main(String[] args) {
// Initialize a string to store the result
String result = "";
// Define two integer arrays
int[] left_array = {1, 3, -5, 4};
int[] right_array = {1, 4, -5, -2};
// Print the elements of Array1
System.out.println("\nArray1: " + Arrays.toString(left_array));
// Print the elements of Array2
System.out.println("\nArray2: " + Arrays.toString(right_array));
// Multiply corresponding elements from both arrays and build the result string
for (int i = 0; i < left_array.length; i++) {
int num1 = left_array[i];
int num2 = right_array[i];
result += Integer.toString(num1 * num2) + " ";
}
// Print the result string
System.out.println("\nResult: " + result);
}
}
Sample Output:
Array1: [1, 3, -5, 4] Array2: [1, 4, -5, -2] Result: 1 12 25 -8
Pictorial Presentation:
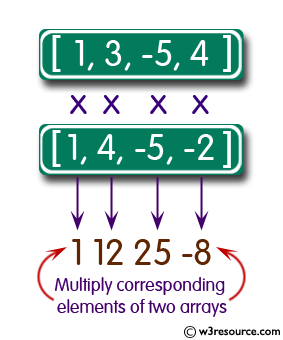
Flowchart:
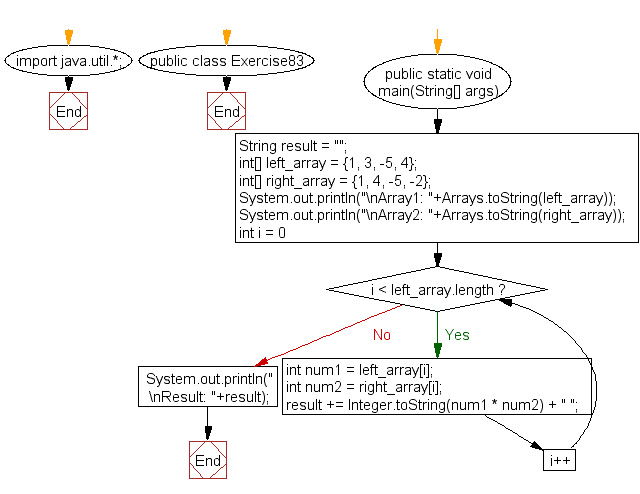
Java Code Editor:
Previous: Write a Java program to find the largest element between first, last, and middle values from an array of integers .
Next: Write a Java program to take the last three characters from a given string and add the three characters at both the front and back of the string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/java-exercises/basic/java-basic-exercise-83.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics