Java: Swap the first and last elements of an array and create a new array
Java Basic: Exercise-81 with Solution
Write a Java program to swap the first and last elements of an array (length must be at least 1) and create another array.
Sample Solution:
Java Code:
import java.util.Arrays;
public class Exercise81 {
public static void main(String[] args) {
// Define an integer array, array_nums
int[] array_nums = {20, 30, 40};
// Print the elements of the original array
System.out.println("Original Array: " + Arrays.toString(array_nums));
// Store the value of the first element in the variable x
int x = array_nums[0];
// Swap the first and last elements of the array
array_nums[0] = array_nums[array_nums.length - 1];
array_nums[array_nums.length - 1] = x;
// Print the new array after swapping the first and last elements
System.out.println("New array after swapping the first and last elements: " + Arrays.toString(array_nums));
}
}
Sample Output:
Original Array: [20, 30, 40] New array after swaping the first and last elements: [40, 30, 20]
Flowchart:
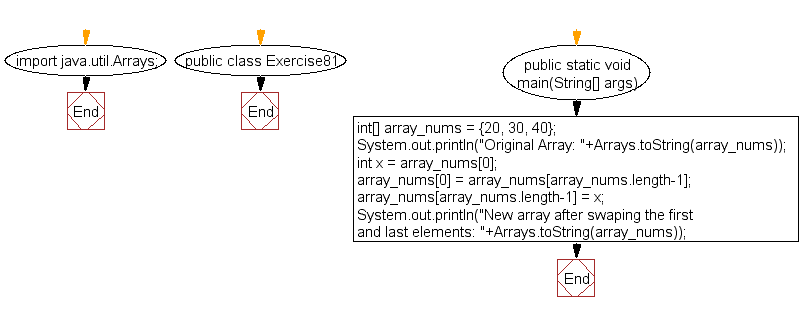
Java Code Editor:
Previous: Write a Java program to get the larger value between first and last element of an array (length 3) of integers.
Next: Write a Java program to find the largest element between first, last, and middle values from an array of integers .
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/java-exercises/basic/java-basic-exercise-81.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics