Java: Get the larger value between first and last element of an array of integers
Largest of First or Last Element
Write a Java program to get the largest value between the first and last elements of an array (length 3) of integers.
Sample Solution:
Java Code:
Sample Output:
Original Array: [20, 30, 40] Larger value between first and last element: 40
Pictorial Presentation:
Flowchart:
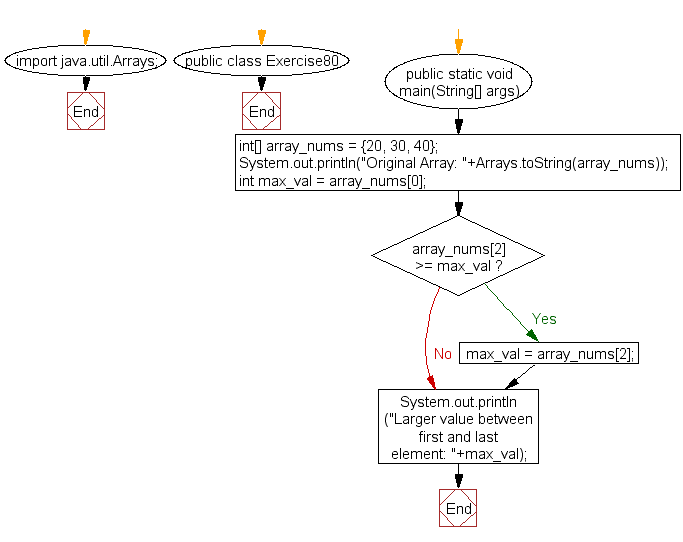
For more Practice: Solve these Related Problems:
- Modify the program to find the smallest element instead.
- Write a program to find the largest of the first, last, and middle elements.
- Modify the program to return the index of the largest element instead of its value.
- Write a program to check if the first or last element is a multiple of 5.
Java Code Editor:
Previous: Write a Java program to rotate an array (length 3) of integers in left direction.
Next: Write a Java program to swap the first and last elements of an array (length must be at least 1) and create a new array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics