Java: Rotate an array of integers in left direction
Rotate Array Left
Write a Java program to rotate an array (length 3) of integers in the left direction.
Test Data: {20, 30, 40}
Expected output: {30, 40, 20}
Sample Solution:
Java Code:
import java.util.Arrays;
public class Exercise79 {
public static void main(String[] args) {
// Define an integer array, array_nums
int[] array_nums = {20, 30, 40};
// Print the elements of the original array
System.out.println("Original Array: " + Arrays.toString(array_nums));
// Create a new array by rotating the elements of the original array
int[] new_array_nums = {array_nums[1], array_nums[2], array_nums[0]};
// Print the elements of the rotated array
System.out.println("Rotated Array: " + Arrays.toString(new_array_nums));
}
}
Sample Output:
Original Array: [20, 30, 40] Rotated Array: [30, 40, 20]
Pictorial Presentation:
Flowchart:
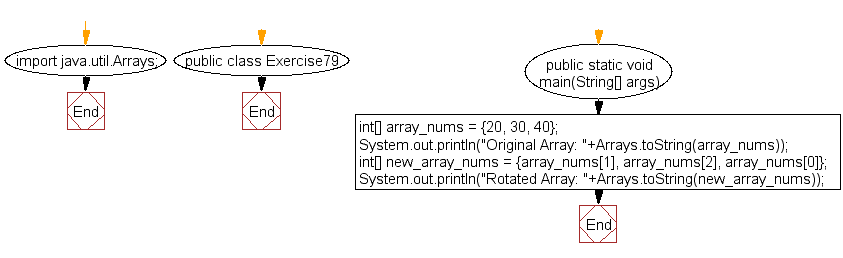
Java Code Editor:
Previous: Write a Java program to test that a given array of integers of length 2 contains a 4 or a 7.
Next: Write a Java program to get the larger value between first and last element of an array (length 3) of integers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics