Java: Test if the first or the last elements of two arrays of integers are same
Java Basic: Exercise-76 with Solution
Write a Java program to test if the first and last element of two integer arrays are the same. The array length must be greater than or equal to 2.
Sample Solution:
Java Code:
public class Main {
public static void main(String[] args) {
// Define two integer arrays (num_array1 and num_array2)
// Example 1: Arrays with different first and last elements
int[] num_array1 = {50, -20, 0, 30, 40, 60, 12};
int[] num_array2 = {45, 20, 10, 20, 30, 50, 11};
// Example 2: Arrays with the same first and last elements
// int[] num_array1 = {50, -20, 0, 30, 40, 60, 50};
// int[] num_array2 = {45, 20, 10, 20, 30, 50, 45};
// Example 3: Arrays with lengths less than 2
// int[] num_array1 = {50};
// int[] num_array2 = {45};
if (num_array1.length >= 2 && num_array2.length >= 2) {
// Check if both arrays have lengths of at least 2 and if their first or last elements are equal
System.out.println(num_array1[0] == num_array2[0] || num_array1[num_array1.length - 1] == num_array2[num_array2.length - 1]);
} else {
System.out.println("Array lengths less than 2.");
}
}
}
Sample Output:
false
Pictorial Presentation:
Flowchart:
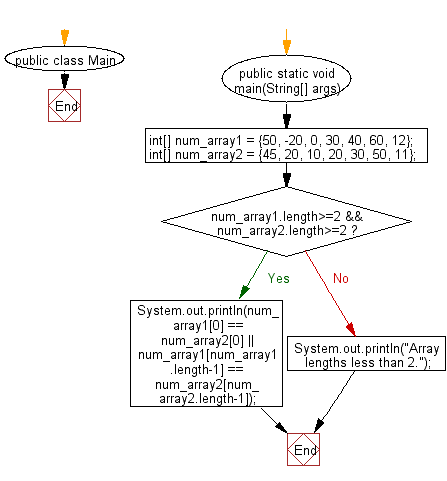
Java Code Editor:
Previous: Write a Java program to test if the first and the last element of an array of integers are same.
Next: Write a Java program to create a new array of length 2 from two arrays of integers with three elements and the new array will contain the first and last elements from the two arrays.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics