Java: Create a string in the form short_string + long_string + short_string from two strings
Short + Long + Short String
Write a Java program to create a string in the form of short_string + long_string + short_string from two strings. The strings must not have the same length.
Pictorial Presentation:
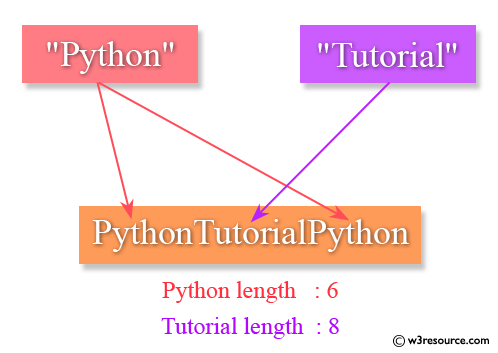
Sample Solution:
Java Code:
import java.lang.*;
public class Exercise70 {
public static void main(String[] args) {
// Define two strings
String str1 = "Python";
String str2 = "Tutorial";
// Check the lengths of the strings
if (str1.length() >= str2.length()) {
// Concatenate the strings in the order: str2 + str1 + str2
System.out.println(str2 + str1 + str2);
} else {
// Concatenate the strings in the order: str1 + str2 + str1
System.out.println(str1 + str2 + str1);
}
}
}
Sample Output:
PythonTutorialPython
Flowchart:
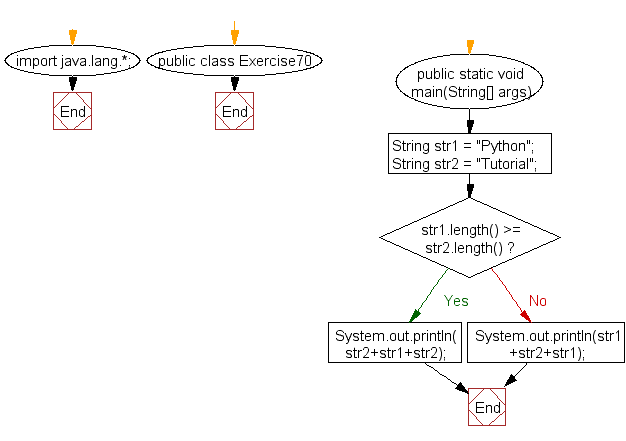
Java Code Editor:
Previous: Write a Java program to extract the first half of a string of even length.
Next: Write a Java program to create the concatenation of the two strings except removing the first character of each string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics