Java: Print multiplication table of a number upto 10
Multiplication Table
Write a Java program that takes a number as input and prints its multiplication table up to 10.
Test Data:
Input a number: 8
Pictorial Presentation:
Sample Solution:
Java Code:
import java.util.Scanner;
public class Exercise7 {
public static void main(String[] args) {
// Create a Scanner object to read input from the user
Scanner in = new Scanner(System.in);
// Prompt the user to input a number
System.out.print("Input a number: ");
// Read and store the input number
int num1 = in.nextInt();
// Use a loop to calculate and print the multiplication table for the input number
for (int i = 0; i < 10; i++) {
// Calculate and print the result of num1 multiplied by (i+1)
System.out.println(num1 + " x " + (i + 1) + " = " + (num1 * (i + 1)));
}
}
}
Explanation:
In the exercise above -
- It takes an integer number as input from the user using the Scanner class.
- It then enters a for loop that iterates 10 times (for values of i from 0 to 9).
- Inside the loop, it calculates and prints the result of multiplying the input number by i+1, displaying a multiplication table for the input number from 1 to 10.
Sample Output:
Input a number: 8 8 x 1 = 8 8 x 2 = 16 8 x 3 = 24 8 x 4 = 32 8 x 5 = 40 8 x 6 = 48 8 x 7 = 56 8 x 8 = 64 8 x 9 = 72 8 x 10 = 80
Flowchart:
Sample Solution:
Java Code:
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
// Create a Scanner object to read input from the user
Scanner in = new Scanner(System.in);
// Prompt the user to input a number
System.out.println("Input the Number: ");
// Read and store the input number
int n = in.nextInt();
// Use a loop to generate and print the multiplication table for the input number
for (int i = 1; i <= 10; i++) {
// Calculate and print the result of n multiplied by i
System.out.println(n + "*" + i + " = " + (n * i));
}
}
}
Sample Output:
Input the Number: 6 6*1 = 6 6*2 = 12 6*3 = 18 6*4 = 24 6*5 = 30 6*6 = 36 6*7 = 42 6*8 = 48 6*9 = 54 6*10 = 60
Flowchart:
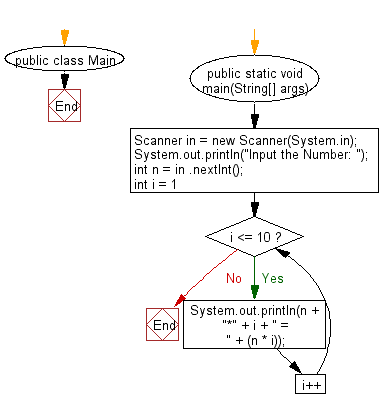
For more Practice: Solve these Related Problems:
- Modify the program to print the multiplication table up to 20 instead of 10.
- Write a program that prints the multiplication table of a given number in reverse order.
- Modify the program to print the multiplication table in a tabular format with proper alignment.
- Write a program that generates the multiplication tables of numbers from 1 to 10.
Go to:
PREV : Basic Arithmetic Operations.
NEXT : Pattern Display: JAVA.
Java Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.