Java: Compute the sum of the prime numbers till 100
Java Basic: Exercise-66 with Solution
Write a Java program to Compute the sum of the prime numbers till 100.
Pictorial Presentation:
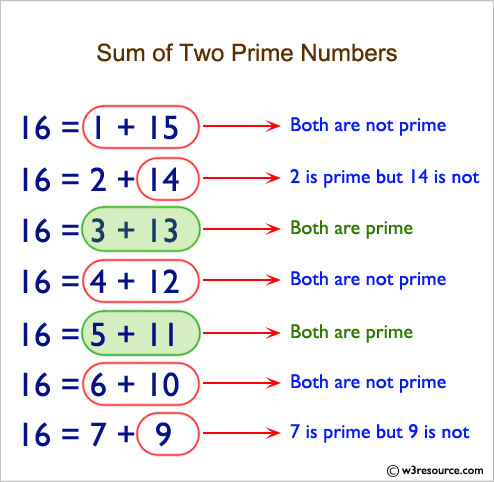
Sample Solution:
Java Code:
import java.util.*;
public class Exercise66 {
public static void main(String[] args) {
// Initialize variables for sum, counter, and the number 'n'
int sum = 1;
int ctr = 0;
int n = 0;
// Iterate while the counter is less than 100
while (ctr < 100) {
n++;
// Check if the number is odd
if (n % 2 != 0) {
// If the number is prime, add it to the sum
if (is_Prime(n)) {
sum += n;
}
}
// Increment the counter
ctr++;
}
// Print the sum of prime numbers up to 100
System.out.println("\nSum of the prime numbers till 100: " + sum);
}
// Check if a number is prime
public static boolean is_Prime(int n) {
for (int i = 3; i * i <= n; i += 2) {
if (n % i == 0) {
return false;
}
}
return true;
}
}
Sample Output:
Sum of the prime numbers till 100: 1060
Flowchart:
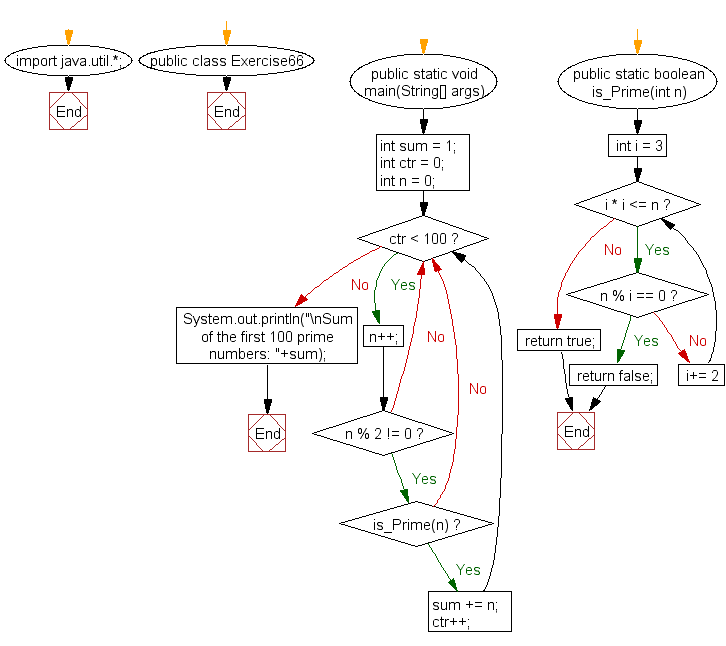
Java Code Editor:
Previous: Write a Java program to calculate the modules of two numbers without using any inbuilt modulus operator.
Next: Write a Java program to insert a word in the middle of the another string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics