Java-: Print the sum, multiply, subtract, divide and remainder of two numbers
Java Basic: Exercise-6 with Solution
Write a Java program to print the sum (addition), multiply, subtract, divide and remainder of two numbers.
Test Data:
Input first number: 125
Input second number: 24
Pictorial Presentation:
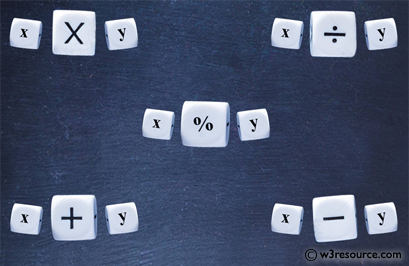
Sample Solution-1
Java Code:
public class Exercise6 {
public static void main(String[] args) {
// Create a Scanner object to read input from the user
Scanner in = new Scanner(System.in);
// Prompt the user to input the first number
System.out.print("Input first number: ");
// Read and store the first number
int num1 = in.nextInt();
// Prompt the user to input the second number
System.out.print("Input second number: ");
// Read and store the second number
// Calculate and print the sum of the two numbers
System.out.println(num1 + " + " + num2 + " = " + (num1 + num2));
// Calculate and print the difference of the two numbers
System.out.println(num1 + " - " + num2 + " = " + (num1 - num2));
// Calculate and print the product of the two numbers
System.out.println(num1 + " x " + num2 + " = " + (num1 * num2));
// Calculate and print the division of the two numbers
System.out.println(num1 + " / " + num2 + " = " + (num1 / num2));
// Calculate and print the remainder of the division of the two numbers
System.out.println(num1 + " mod " + num2 + " = " + (num1 % num2);
}
}
Explanation:
In the exercise above -
- It takes two integer numbers as input from the user using the Scanner class.
- Scanner in = new Scanner(System.in);
- System.out.print("Input first number: ");
- int num1 = in.nextInt();
- System.out.print("Input second number: ");
- System.out.println(num1 + " + " + num2 + " = " + (num1 + num2)); - It calculates and displays the sum of the two numbers.
- System.out.println(num1 + " - " + num2 + " = " + (num1 - num2)); - It calculates and displays the difference between the two numbers.
- System.out.println(num1 + " x " + num2 + " = " + (num1 * num2)); - It calculates and displays the product of the two numbers.
- System.out.println(num1 + " / " + num2 + " = " + (num1 / num2)); - It calculates and displays the result of dividing the first number by the second number.
- System.out.println(num1 + " mod " + num2 + " = " + (num1 % num2)); - It calculates and displays the remainder (modulus) when the first number is divided by the second number.
Sample Output:
Input first number: 125 Input second number: 24 125 + 24 = 149 125 - 24 = 101 125 x 24 = 3000 125 / 24 = 5 125 mod 24 = 5
Flowchart:
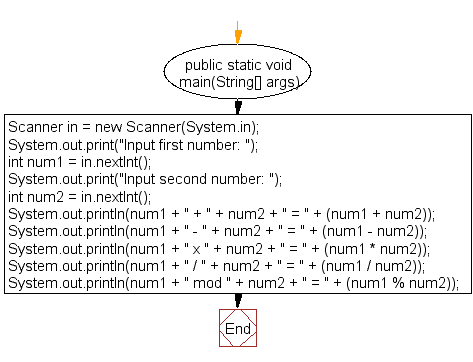
Sample Solution-2
Java Code:
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
// Create a Scanner object to read input from the user
Scanner scanner = new Scanner(System.in);
// Prompt the user to input the first number
System.out.println("Input the first number: ");
// Read and store the first number
int n1 = scanner.nextInt();
// Prompt the user to input the second number
System.out.println("Input the second number: ");
// Read and store the second number
// Calculate the sum of the two numbers
int sum = n1 + n2;
// Calculate the difference of the two numbers
int minus = n1 - n2;
// Calculate the product of the two numbers
int multiply = n1 * n2;
// Calculate the addition of the two numbers (Note: This comment may be a typo; it seems similar to the "sum" calculation)
int subtract = n1 + n2;
// Calculate the division of the two numbers
int divide = n1 / n2;
// Calculate the remainder when dividing the two numbers
int rnums = n1 % n2;
// Display the results of the calculations
System.out.printf("Sum = %d\nMinus = %d\nMultiply = %d\nSubtract = %d\nDivide = %d\nRemainderOf2Numbers = %d\n ", sum, minus, multiply, subtract, divide, rnums);
}
}
Sample Output:
Input the first number: 6 Input the second number: 5 Sum = 11 Minus = 1 Multiply = 30 Subtract = 11 Divide = 1 RemainderOf2Numbers = 1
Flowchart:
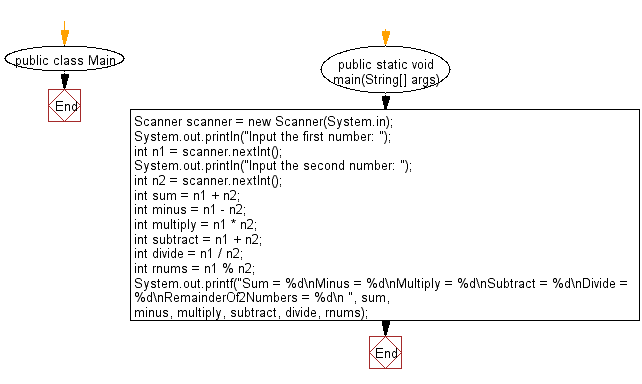
Java Code Editor:
Previous: Write a Java program that takes two numbers as input and display the product of two numbers.
Next: Write a Java program that takes a number as input and prints its multiplication table upto 10.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/java-exercises/basic/java-basic-exercise-6.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics