Java: Convert seconds to hour, minute and seconds
Java Basic: Exercise-55 with Solution
Write a Java program to convert seconds to hours, minutes and seconds.
Pictorial Presentation:
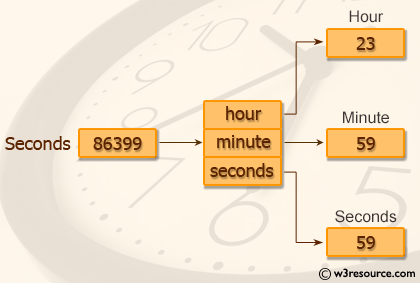
Sample Data:
Input seconds: 86399
23:59:59
Sample Solution:
Java Code:
import java.util.*;
public class Main {
public static void main(String[] args) {
// Create a Scanner object for user input
Scanner in = new Scanner(System.in);
// Prompt the user to input the total seconds
System.out.print("Input seconds: ");
int seconds = in.nextInt();
// Calculate the hours, minutes, and seconds
int S = seconds % 60; // Calculate the remaining seconds
int H = seconds / 60; // Convert total seconds to minutes
int M = H % 60; // Calculate the remaining minutes
H = H / 60; // Convert total minutes to hours
// Display the time in the format HH:MM:SS
System.out.print(H + ":" + M + ":" + S);
// Print a new line for better formatting
System.out.print("\n");
}
}
Sample Output:
Input seconds: 86399 23:59:59
Pictorial Presentation:
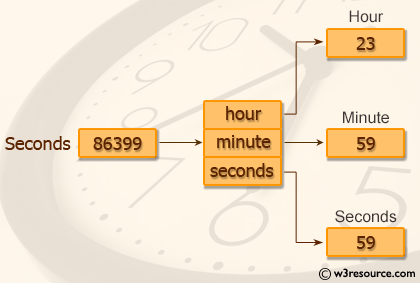
Flowchart:
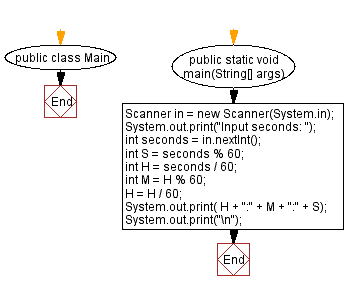
Java Code Editor:
Previous: Write a Java program that accepts three integers from the user and return true if two or more of them (integers ) have the same rightmost digit.
Next: Write a Java program to find the number of values in a given range divisible by a given value.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics