Java: Convert a string to an integer
String to Integer Conversion
Write a Java program to convert a string to an integer.
Sample Solution:
Java Code:
import java.util.*;
public class Exercise51 {
public static void main(String[] args) {
// Create a Scanner object for user input
Scanner in = new Scanner(System.in);
// Prompt the user to input a number (as a string)
System.out.print("Input a number (string): ");
// Read the input string and store it in str1
String str1 = in.nextLine();
// Parse the string as an integer
int result = Integer.parseInt(str1);
// Display the integer value
System.out.printf("The integer value is: %d", result);
// Print a new line for better formatting
System.out.printf("\n");
}
}
Sample Output:
Input a number(string): 25 The integer value is: 25
Flowchart:
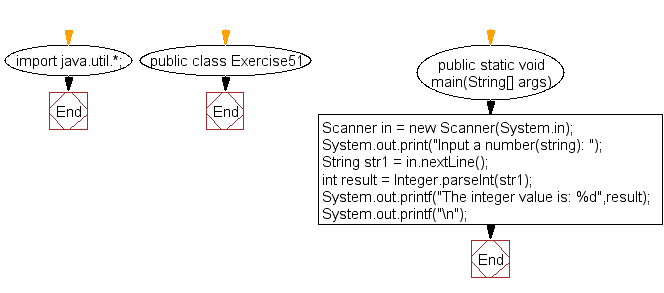
Java Code Editor:
Previous: Write a Java program to print numbers between 1 to 100 which are divisible by 3, 5 and by both.
Next: Write a Java program to calculate the sum of two integers and return true if the sum is equal to a third integer.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics