Java: Accept a number and check the number is even or not
Java Basic: Exercise-49 with Solution
Write a Java program to accept a number and check whether the number is even or not. Prints 1 if the number is even or 0 if odd.
Pictorial Presentation:
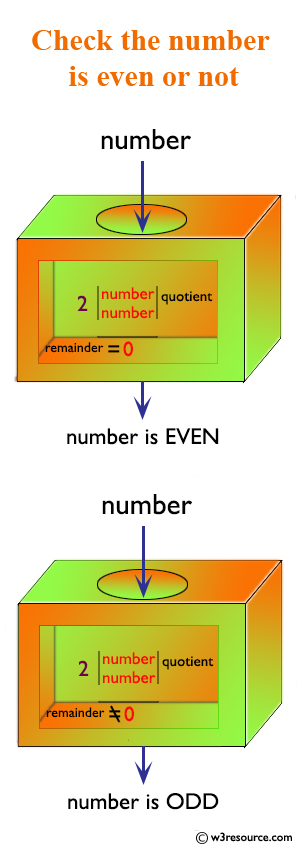
Sample Solution:
Java Code:
import java.util.*;
public class Exercise49 {
public static void main(String[] args) {
// Create a scanner for user input
Scanner in = new Scanner(System.in);
// Prompt the user to input a number
System.out.print("Input a number: ");
int n = in.nextInt();
// Check if the number is even and print the result
if (n % 2 == 0) {
System.out.println(1); // If the number is even, print 1
} else {
System.out.println(0); // If the number is odd, print 0
}
}
}
Sample Output:
Input a number: 20 1
Flowchart:
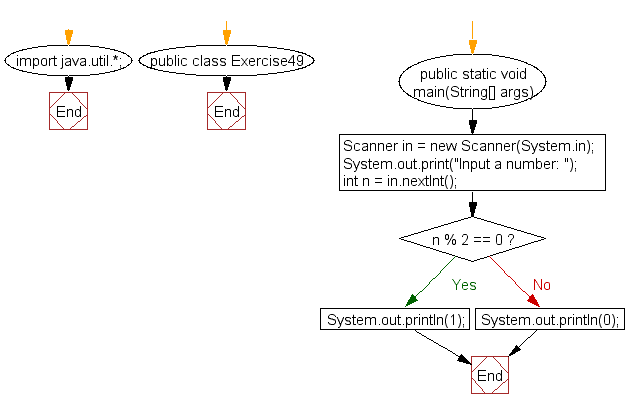
Java Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Java program to print the odd numbers from 1 to 99. Prints one number per line.
Next: Write a Java program to print numbers between 1 to 100 which are divisible by 3, 5 and by both.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics