Java: List the available character sets in charset objects
Java Basic: Exercise-40 with Solution
Write a Java program to list the available character sets in charset objects.
Sample Solution:
Java Code:
import java.nio.charset.Charset;
public class Exercise40 {
public static void main(String[] args) {
System.out.println("List of available character sets: ");
// Iterate through the available character sets and print their names
for (String str : Charset.availableCharsets().keySet()) {
System.out.println(str);
}
}
}
Explanation:
In the exercise above -
- First, import the Charset class from the "java.nio.charset" package.
- In the "main()" method, it prints the header "List of available character sets."
- It uses a for-each loop to iterate through the keys (character set names) in the set of available character sets provided by Charset.availableCharsets().keySet().
- Inside the loop, it prints each character set name on a separate line.
- Finally, when you run the program, it will list and display the names of all available character sets (encodings) supported by your Java runtime environment.
Sample Output:
List of available character sets: Big5 Big5-HKSCS CESU-8 EUC-JP EUC-KR GB18030 GB2312 GBK IBM-Thai IBM00858 IBM01140 ----- x-windows-950 x-windows-iso2022jp
Flowchart:
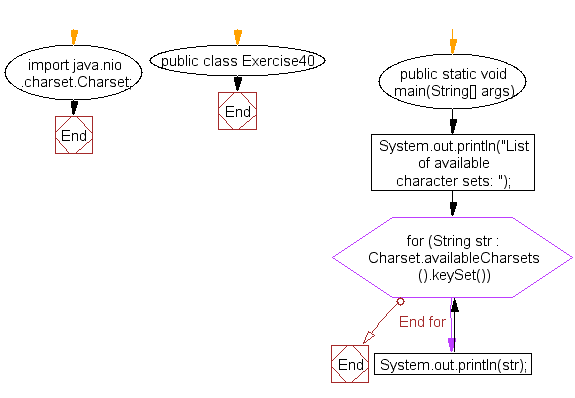
Java Code Editor:
Previous: Write a Java program to create and display unique three-digit number using 1, 2, 3, 4. Also count how many three-digit numbers are there.
Next: Write a Java program to print the ascii value of a given character.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics