Java: Compute the distance between two points on the surface of earth
Distance Between Two Points
Write a Java program to compute the distance between two points on the earth's surface.
Distance between the two points [ (x1,y1) & (x2,y2)]
d = radius * arccos(sin(x1) * sin(x2) + cos(x1) * cos(x2) * cos(y1 - y2))
Radius of the earth r = 6371.01 Kilometers
Test Data:
Input the latitude of coordinate 1: 25
Input the longitude of coordinate 1: 35
Input the latitude of coordinate 2: 52.5
Input the longitude of coordinate 2: 35.5
Pictorial Presentation:
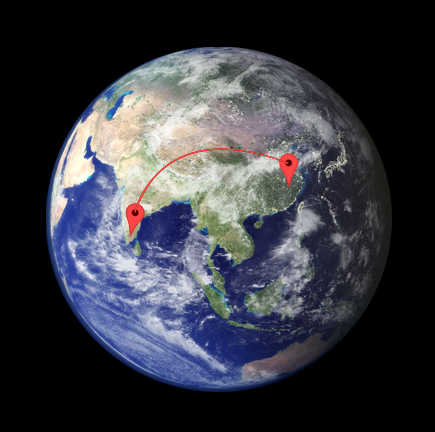
Sample Solution:
Java Code:
import java.util.Scanner;
public class Exercise36 {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
// Prompt the user to input the latitude and longitude of coordinate 1
System.out.print("Input the latitude of coordinate 1: ");
double lat1 = input.nextDouble();
System.out.print("Input the longitude of coordinate 1: ");
double lon1 = input.nextDouble();
// Prompt the user to input the latitude and longitude of coordinate 2
System.out.print("Input the latitude of coordinate 2: ");
double lat2 = input.nextDouble();
System.out.print("Input the longitude of coordinate 2: ");
double lon2 = input.nextDouble();
// Calculate and display the distance between the two coordinates
System.out.print("The distance between those points is: " + distance_Between_LatLong(lat1, lon1, lat2, lon2) + " km\n");
}
// Points will be converted to radians before calculation
public static double distance_Between_LatLong(double lat1, double lon1, double lat2, double lon2) {
// Convert latitude and longitude to radians
lat1 = Math.toRadians(lat1);
lon1 = Math.toRadians(lon1);
lat2 = Math.toRadians(lat2);
lon2 = Math.toRadians(lon2);
// Earth's mean radius in kilometers
double earthRadius = 6371.01;
// Calculate the distance using the haversine formula
return earthRadius * Math.acos(Math.sin(lat1) * Math.sin(lat2) + Math.cos(lat1) * Math.cos(lat2) * Math.cos(lon1 - lon2));
}
}
Explanation:
In the exercise above -
- First, it uses the "Scanner" class to obtain user input.
- The user is prompted to input the latitude and longitude of two coordinates:
- Latitude and longitude of coordinate 1 are read into the variables 'lat1' and 'lon1'.
- Latitude and longitude of coordinate 2 are read into the variables 'lat2' and 'lon2'.
- The code then calls a separate method named "distance_Between_LatLong()" and passes the latitude and longitude values of both coordinates as arguments.
- Inside the "distance_Between_LatLong()" method:
- It converts latitude and longitude values from degrees to radians using the "Math.toRadians()" method to perform trigonometric calculations.
- It calculates the distance between the two coordinates using the Haversine formula for calculating distances on a sphere.
- The calculated distance is returned as a double, representing the distance in kilometers.
- Finally, in the "main() method, the result of the "distance_Between_LatLong()" method is printed, displaying the distance between the two coordinates in kilometers.
Sample Output:
Input the latitude of coordinate 1: 25 Input the longitude of coordinate 1: 35 Input the latitude of coordinate 2: 52.5 Input the longitude of coordinate 2: 35.5 The distance between those points is: 3058.15512920181 km
Flowchart:
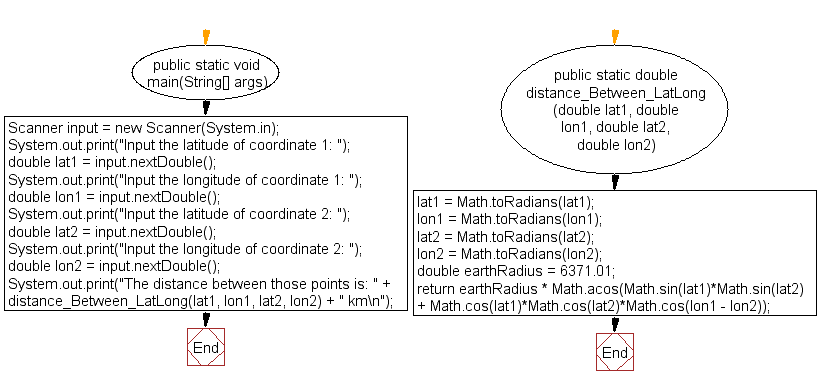
For more Practice: Solve these Related Problems:
- Modify the program to work with miles instead of kilometers.
- Write a program that finds the midpoint between two coordinates.
- Compute the distance between three points instead of two.
- Modify the program to calculate the Manhattan distance.
Java Code Editor:
Previous: Write a Java program to compute the area of a polygon.
Next: Write a Java program to reverse a string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics