Java: Check whether Java is installed on your computer
Java Basic: Exercise-31 with Solution
Write a Java program to check whether Java is installed on your computer.
Pictorial Presentation:
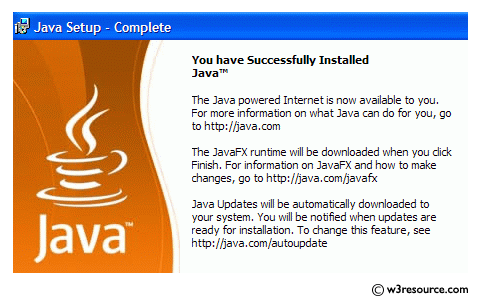
Sample Solution:
Java Code:
public class Exercise31 {
public static void main(String[] args) {
// Display Java version
System.out.println("\nJava Version: " + System.getProperty("java.version"));
// Display Java runtime version
System.out.println("Java Runtime Version: " + System.getProperty("java.runtime.version"));
// Display Java home directory
System.out.println("Java Home: " + System.getProperty("java.home"));
// Display Java vendor name
System.out.println("Java Vendor: " + System.getProperty("java.vendor"));
// Display Java vendor URL
System.out.println("Java Vendor URL: " + System.getProperty("java.vendor.url"));
// Display Java class path
System.out.println("Java Class Path: " + System.getProperty("java.class.path") + "\n");
}
}
Explanation:
In the exercise above:
- It uses System.getProperty() to access and retrieve specific Java system properties.
- Finally, it prints the following information about the Java runtime environment:
- Java Version: The Java version installed on the system.
- Java Runtime Version: The Java Runtime Environment (JRE) used.
- Java Home: The directory path to the Java installation directory.
- Java Vendor: The Java runtime vendor or provider.
- Java Vendor URL: The Java vendor's URL or website.
- Java Class Path: The classpath, which specifies the directories and JAR files that the Java runtime should use when searching for classes and resources.
Sample Output:
Java Version: 1.8.0_71 Java Runtime Version: 1.8.0_71-b15 Java Home: /opt/jdk/jdk1.8.0_71/jre Java Vendor: Oracle Corporation Java Vendor URL: http://java.oracle.com/ Java Class Path: .
Flowchart:
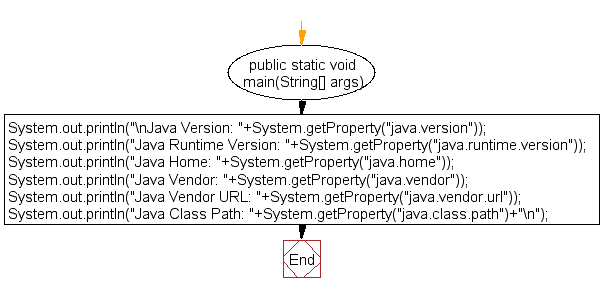
Java Code Editor:
Previous: Write a Java program to convert a hexadecimal to a octal number.
Next: Write a Java program to compare two numbers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics