Java: Convert a hexadecimal to a octal number
Java Basic: Exercise-30 with Solution
Write a Java program to convert a hexadecimal value into an octal number.
Hexadecimal number: This is a positional numeral system with a radix, or base, of 16. Hexadecimal uses sixteen distinct symbols, most often the symbols 0-9 to represent values zero to nine, and A, B, C, D, E, F (or alternatively a, b, c, d, e, f) to represent values ten to fifteen.
Octal number: The octal numeral system is the base-8 number system, and uses the digits 0 to 7.
Test Data:
Input any hexadecimal number: 40
Pictorial Presentation: Hexadecimal to Octal number
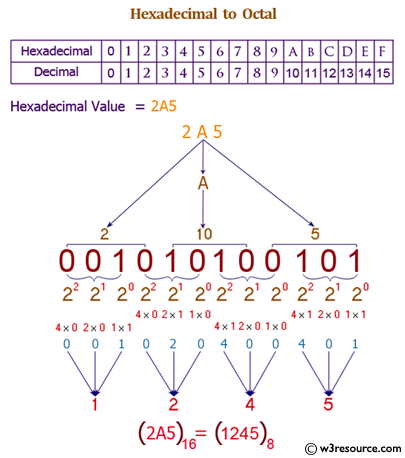
Sample Solution:
Java Code:
import java.util.Scanner;
public class Main {
// Function to convert a hexadecimal string to a decimal integer
public static int hex_to_oct(String s) {
// Define a string containing hexadecimal digits
String digits = "0123456789ABCDEF";
s = s.toUpperCase(); // Convert the input string to uppercase
int val = 0; // Initialize the decimal value to 0
// Iterate through each character in the input string
for (int i = 0; i < s.length(); i++) {
char c = s.charAt(i); // Get the current character
int d = digits.indexOf(c); // Find the index of the character in the digits string
val = 16 * val + d; // Update the decimal value using hexadecimal conversion
}
return val; // Return the decimal value
}
public static void main(String args[]) {
String hexdec_num;
int dec_num, i = 1, j;
int octal_num[] = new int[100];
Scanner in = new Scanner(System.in);
// Prompt the user to input a hexadecimal number
System.out.print("Input a hexadecimal number: ");
hexdec_num = in.nextLine();
// Call the hex_to_oct function to convert the hexadecimal number to decimal
dec_num = hex_to_oct(hexdec_num);
// Convert the decimal number to octal
while (dec_num != 0) {
octal_num[i++] = dec_num % 8;
dec_num = dec_num / 8;
}
// Display the equivalent octal number
System.out.print("Equivalent of octal number is: ");
for (j = i - 1; j > 0; j--) {
System.out.print(octal_num[j]);
}
System.out.print("\n");
}
}
Explanation:
In the exercise above -
- First, it defines a method "hex_to_oct()" that takes a hexadecimal string 's' as input and converts it to its decimal equivalent. It initializes a string 'digits' containing the hexadecimal digits (0-9 and A-F), ensures that the input string 's' is in uppercase, and iterates through the characters to compute the decimal value.
- In the "main()" function, it takes a hexadecimal number as input from the user using the "Scanner" class and stores it in the 'hexdec_num' string.
- It calls the " hex_to_oct ()" method, passing 'hexdec_num' as an argument, to convert the hexadecimal string to its decimal equivalent and stores it in the 'dec_num' variable.
- Then, it converts the decimal number to octal:
- It initializes an integer array 'octal_num' to store the octal digits.
- It enters a loop to perform the decimal-to-octal conversion:
- In each iteration, it calculates the remainder when 'dec_num' is divided by 8 (which gives the least significant octal digit).
- It stores this remainder in the 'octal_num' array.
- It updates 'dec_num' by dividing it by 8 to move to the next octal digit.
- It uses 'i' to keep track of each octal digit's position.
- Finally, it prints the octal representation of the original hexadecimal number by iterating through the 'octal_num' array in reverse order (from the most significant digit to the least significant digit).
Sample Output:
Input a hexadecimal number: 40 Equivalent of octal number is: 100
Flowchart:
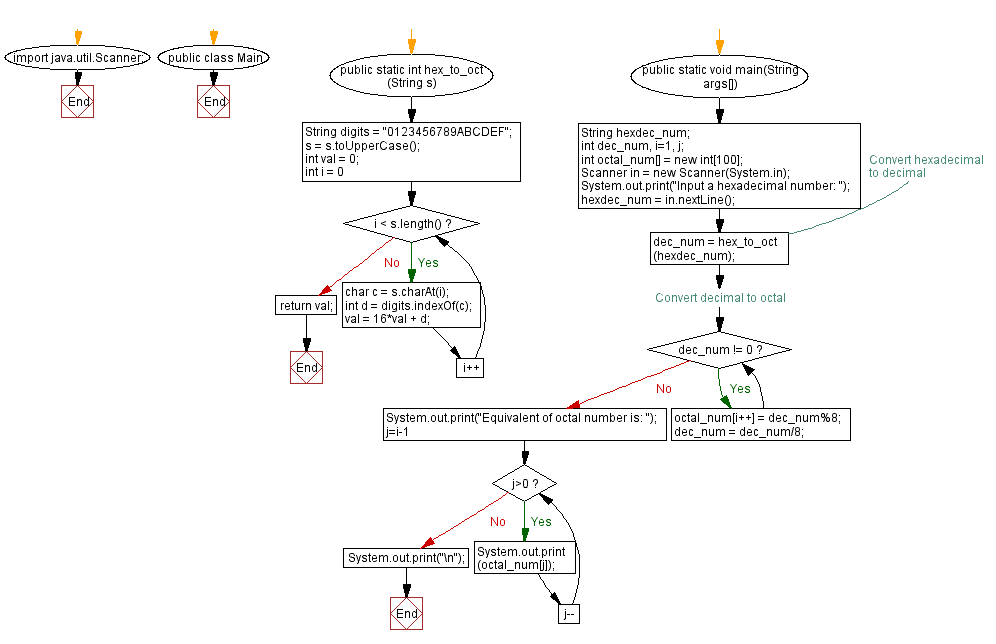
Java Code Editor:
Previous: Write a Java program to convert a hexadecimal to a binary number.
Next: Write a Java program to check whether Java is installed on your computer.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/java-exercises/basic/java-basic-exercise-30.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics