Java: Convert 3 digits positive number in given format
Convert 3-Digit Numbers to "HHTTT123" Format
Let us use the letter H to mean "hundred", the letter T to mean "ten" and “1, 2, . . . n” to represent the one digit n (<10). Using the given format, write a Java program that converts 3 digits positive numbers. For example, 234 should be output as BBSSS1234 because it has 2 "hundreds", 3 "ten", and 4 ones.
Input:
235
230
Output:
HHTTT12345
HHTTT
Visual Presentation:
Sample Solution:
Java Code:
// Importing Scanner class for user input
import java.util.Scanner;
// Defining a class named "Main"
public class Main {
// Main method, the entry point of the program
public static void main(String[] args) {
// Creating a Scanner object for reading user input
Scanner in = new Scanner(System.in);
// Prompting the user to input a positive number with a maximum of three digits
System.out.println("Input a positive number(max three digits):");
// Reading an integer, formatting it as a three-digit string, and converting it to a character array
char[] num = String.format("%03d", in.nextInt()).toCharArray();
// Creating a StringBuilder object to build the result string
StringBuilder tm = new StringBuilder();
// Appending "H" to the StringBuilder based on the hundreds digit of the input number
for (int i = 0; i < num[0] - '0'; i++) {
tm.append("H");
}
// Appending "T" to the StringBuilder based on the tens digit of the input number
for (int i = 0; i < num[1] - '0'; i++) {
tm.append("T");
}
// Appending numbers from 1 to the units digit to the StringBuilder
for (int i = 0; i < num[2] - '0'; i++) {
tm.append(i + 1);
}
// Printing the result
System.out.println("Result:");
System.out.println(tm.toString());
}
}
Sample Output:
Input a positive number(max three digits): 235 Result: HHTTT12345
Flowchart:
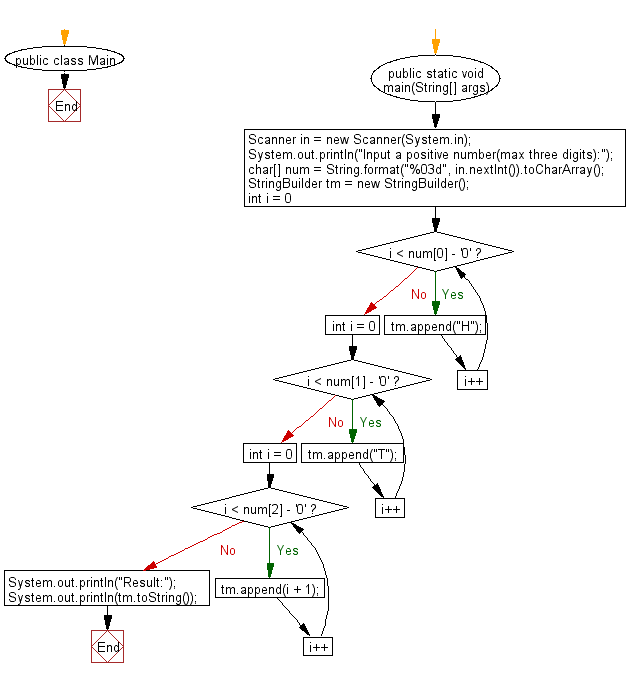
Java Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Java program which accepts students name, id, and marks and display the highest score and the lowest score.
Next: Write a Java program which accepts three integers and check whether sum of the first two given integers is greater than third one. Three integers are in the interval [-231, 231 ].
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics