Java: Reads a list of pairs of a word and a page number, and prints the word and a list of the corresponding page numbers
Pair Words with Page Numbers
Write a Java program that reads a list of pairs of a word and a page number. It prints the words and a list of page numbers.
The number of pairs of a word and a page number is less than or equal to 1000. A word never appear in a page more than once. The words should be printed in alphabetical order and the page numbers should be printed in ascending order.
Visual Presentation:
Input:
Input pairs of a word and a page number:
apple 5
banana 6
Word and page number in alphabetical order:
apple
5
banana
6
Sample Solution:
Java Code:
import java.util.PriorityQueue;
import java.util.Scanner;
public class Main {
// Nested static class named "Dic" implementing Comparable interface
static class Dic implements Comparable<Dic> {
String moji; // Instance variable to store a word
int page; // Instance variable to store a page number
// Constructor to initialize the instance variables
Dic(String moji, int page) {
this.moji = moji;
this.page = page;
}
// Overriding the compareTo method to define the natural ordering of Dic objects
public int compareTo(Dic d) {
// Comparing based on the word, then on the page number if words are equal
if (this.moji.equals(d.moji)) {
return this.page - d.page;
} else {
return this.moji.compareTo(d.moji);
}
}
}
// Main method, the entry point of the program
public static void main(String[] args) {
// Using try-with-resources to automatically close the Scanner
try (Scanner sc = new Scanner(System.in)) {
// Creating a PriorityQueue to store Dic objects
PriorityQueue<Dic> pq = new PriorityQueue<>();
// Prompting the user to input pairs of a word and a page number
System.out.println("Input pairs of a word and a page number (type 'exit' to end input):");
// Loop to read input until there are no more lines
while (sc.hasNextLine()) {
// Reading a line of input and splitting it into word and page number
String str = sc.nextLine();
// Check for the sentinel value to exit the loop
if (str.equals("exit")) {
break;
}
// Splitting the input line into an array of tokens
String[] token = str.split(" ");
// Extracting the word and page number from the tokens
String s = token[0];
int n = Integer.parseInt(token[1]);
// Adding a new Dic object to the PriorityQueue
pq.add(new Dic(s, n));
}
// Initializing a variable to store the previous word
String pre = "";
// Printing the header for the output
System.out.println("\nWord and page number in alphabetical order:");
// Loop to process and print the PriorityQueue
while (!pq.isEmpty()) {
// Polling the head of the PriorityQueue (smallest Dic object)
Dic dic = pq.poll();
// Checking if the current word is the same as the previous one
if (dic.moji.equals(pre)) {
// Printing the page number without a newline and a space
System.out.print(" " + dic.page);
} else if (pre.equals("")) {
// Printing the word and the page number without a newline
System.out.println(dic.moji);
System.out.print(dic.page);
} else {
// Printing a newline, the word, and the page number without a newline
System.out.println();
System.out.println(dic.moji);
System.out.print(dic.page);
}
// Updating the previous word
pre = dic.moji;
}
// Printing a newline at the end of the output
System.out.println();
}
}
}
Sample Output:
Input pairs of a word and a page number: apple 5 banana 6 Word and page number in alphabetical order: apple 5 banana 6
Flowchart:
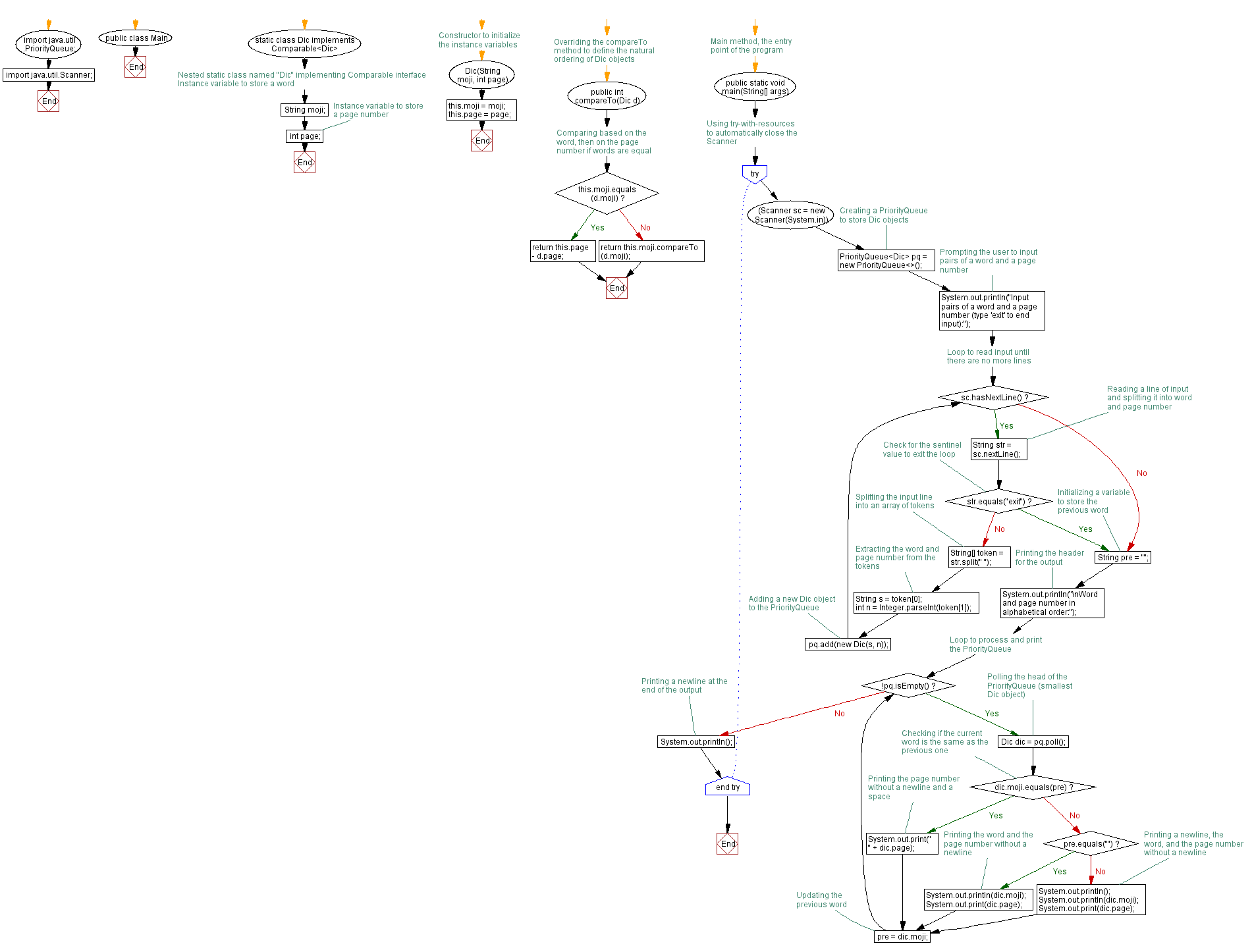
Flowchart:
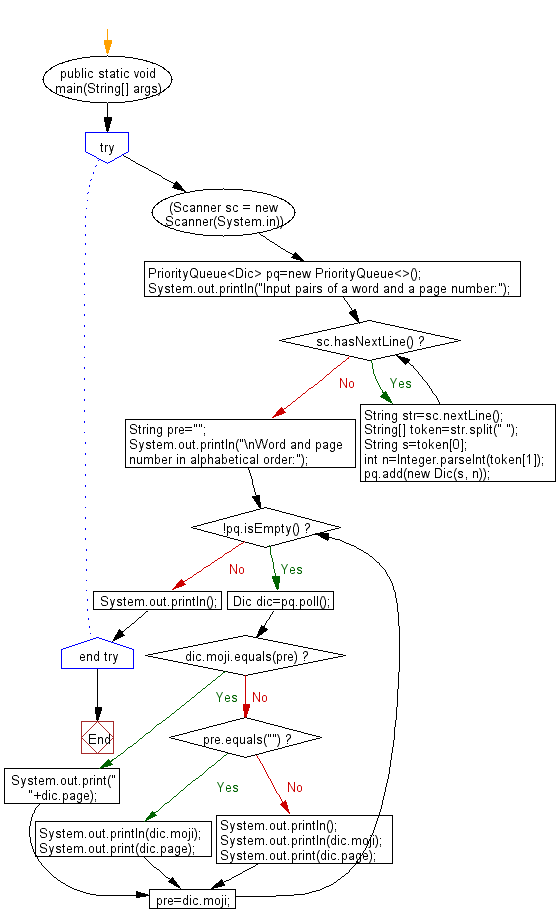
Java Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Java program which adds up columns and rows of given table as shown in the specified figure.
Next: Write a Java program which accepts a string from the user and check whether the string is correct or not.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics