Java: Test whether AB and CD are orthogonal or not
Java Basic: Exercise-235 with Solution
There are four different points on a plane: P(xp, yp), Q(xq, yq), R(xr, yr) and S(xs, ys).
Write a Java program to test whether AB and CD are orthogonal or not.
Input:
xp,yp, xq, yq, xr, yr, xs and ys are -100 to 100 respectively and each value can be up to 5 digits after the decimal point It is given as a real number including the number of.
Output: Yes or No.
Visual Presentation:
Sample Solution:
Java Code:
// Importing the necessary package for Scanner class
import java.util.*;
// Importing the static Math class for using static methods
import static java.lang.Math.*;
// Main class named "Main"
class Main{
// Main method to execute the program
public static void main(String args[]){
// Prompting the user to input coordinates
System.out.println("Input xp, yp, xq, yq, xr, yr, xs, ys:");
// Creating a Scanner object to read input from the console
Scanner scan = new Scanner(System.in);
// Arrays to store x and y coordinates
double x[] = new double[4];
double y[] = new double[4];
// Reading input for coordinates
for(int i=0;i<4;i++){
x[i] = scan.nextDouble();
y[i] = scan.nextDouble();
}
// Calculating the product of differences for x and y coordinates
double a = (x[0] - x[1]) * (x[2] - x[3]);
double b = (y[0] - y[1]) * (y[2] - y[3]);
// Checking if the sum of products is zero to determine orthogonality
if((float)a + (float)b == 0)
System.out.println("Two lines are orthogonal.");
else
System.out.println("Two lines are not orthogonal.");
}
}
Sample Output:
Input xp, yp, xq, yq, xr, yr, xs, ys: 3.5 4.5 2.5 -1.5 3.5 1.0 0.0 4.5 Two lines are not orthogonal.
Flowchart:
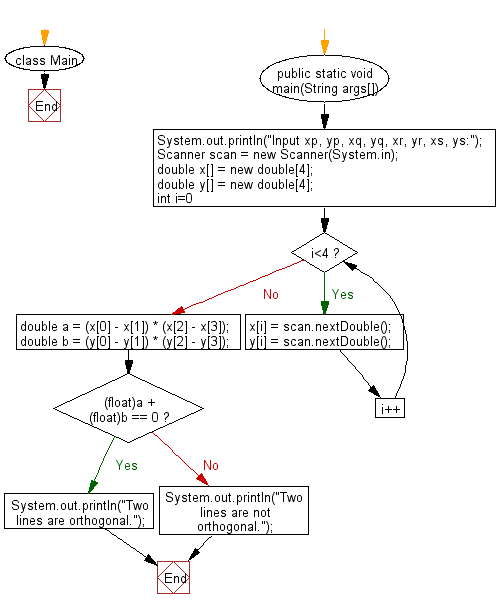
Java Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Java program to create maximum number of regions obtained by drawing n given straight lines.
Next: Write a Java program to sum of all numerical values (positive integers) embedded in a sentence.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics