Java: Test if circumference of two circles intersect or overlap
Java Basic: Exercise-224 with Solution
There are two circles C1 with radius r1, central coordinate (x1, y1) and C2 with radius r2 and central coordinate (x2, y2).
Write a Java program to test the followings -
"C2 is in C1" if C2 is in C1
"C1 is in C2" if C1 is in C2
"Circumference of C1 and C2 intersect" if circumference of C1 and C2 intersect, and
"C1 and C2 do not overlap" if C1 and C2 do not overlap.
Input:
Input numbers (real numbers) are separated by a space.
Visual Presentation:
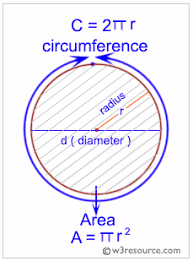
Sample Solution:
Java Code:
// Importing the Scanner class for user input
import java.util.Scanner;
// Main class named "Main"
public class Main {
// Main method to execute the program
public static void main(String arg[]) {
// Creating a Scanner object for user input
Scanner in = new Scanner(System.in);
// Prompting the user to input x1, y1, and r1 for the first circle
System.out.println("Input x1, y1, r1: (numbers are separated by a space)");
double x1 = in.nextDouble(), y1 = in.nextDouble(), r1 = in.nextDouble();
// Prompting the user to input x2, y2, and r2 for the second circle
System.out.println("Input x2, y2, r2: (numbers are separated by a space)");
double x2 = in.nextDouble(), y2 = in.nextDouble(), r2 = in.nextDouble();
// Calculating the distance between the centers of the two circles
double l = Math.sqrt((x1 - x2) * (x1 - x2) + (y1 - y2) * (y1 - y2));
// Checking the relationship between the circles based on their radii and distance
if (l > r1 + r2)
System.out.println("Circumference of C1 and C2 intersect");
else if (r1 > l + r2)
System.out.println("C2 is in C1");
else if (r2 > l + r1)
System.out.println("C1 is in C2");
else
System.out.println("C1 and C2 do not overlap");
}
}
Sample Output:
Input x1, y1, r1: (numbers are separated by a space) 5 6 8 7 Input x2, y2, r2: (numbers are separated by a space) 8 9 5 4 C1 and C2 do not overlap
Flowchart:
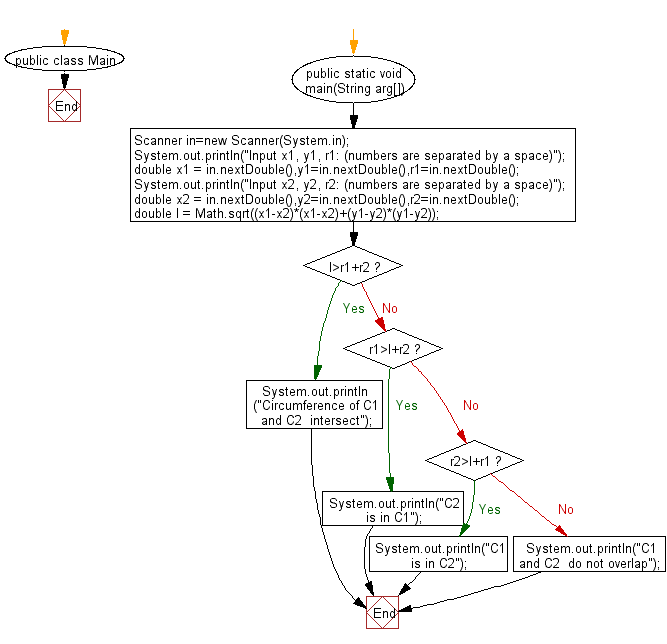
Java Code Editor:
Contribute your code and comments through Disqus.
Previous:Write a Java program to find the maximum sum of a contiguous subsequence from a given sequence of numbers a1, a2, a3, ... an. A subsequence of one element is also a continuous subsequence.
Next: Write a Java program to that reads a date (from 2004/1/1 to 2004/12/31) and prints the day of the date. Jan. 1, 2004, is Thursday. Note that 2004 is a leap year.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics