Java: Print the number of prime numbers which are less than or equal to a given integer
Java Basic: Exercise-217 with Solution
Write a Java program to print the number of prime numbers less than or equal to a given integer.
Input:
n (1 ≤ n ≤ 999,999).
Visual Presentation:
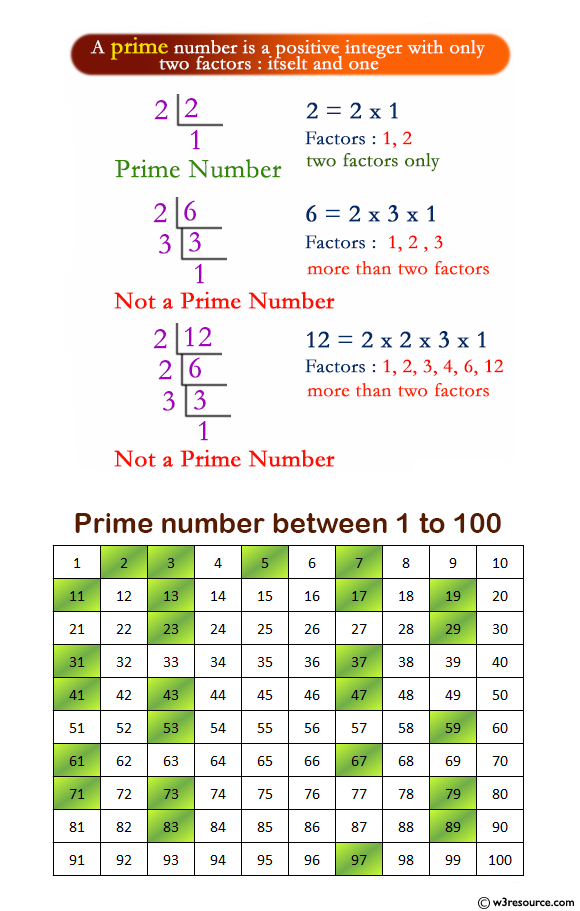
Sample Solution-1:
Java Code:
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
// Prompting the user to input the number (n)
System.out.println("Input the number(n):");
// Creating a Scanner object for user input
Scanner s = new Scanner(System.in);
// Reading the input number (n) from the user
int c = s.nextInt();
// Calling the check method to find the number of prime numbers
int ans = check(c);
// Displaying the number of prime numbers which are less than or equal to n
System.out.println("Number of prime numbers which are less than or equal to n:");
System.out.println(ans);
}
// Method to check the number of prime numbers
static int check(int c) {
// Creating a boolean array to mark numbers as prime or not
boolean[] prime = new boolean[c + 1];
// Initializing a counter for prime numbers
int count = 0;
// Loop to mark non-prime numbers in the array
for (int i = 2; i <= Math.sqrt(c); i++) {
for (int j = i + i; j <= c; j += i) {
prime[j] = true;
}
}
// Counting the number of prime numbers
for (int i = 2; i <= c; i++) {
if (!prime[i]) {
count++;
}
}
// Returning the total number of prime numbers
return count;
}
}
Sample Output:
Input the number(n): 1235 Number of prime numbers which are less than or equal to n.: 202
Flowchart:
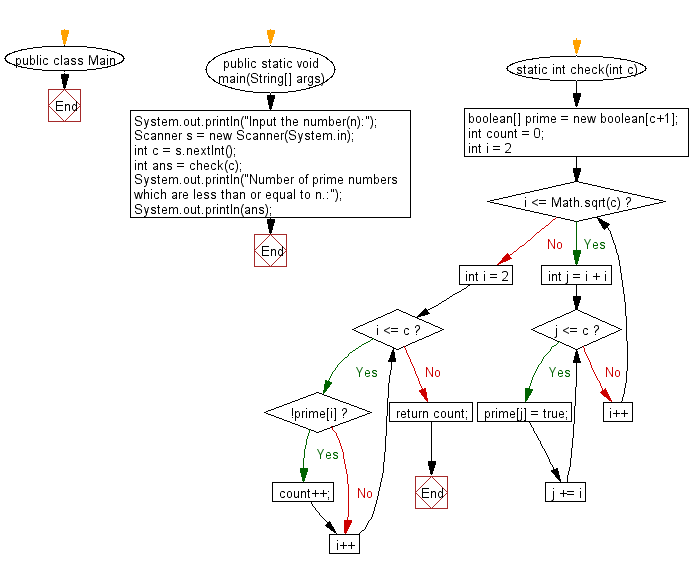
Sample Solution-2:
Java Code:
// Importing the Scanner class to read user input
import java.util.Scanner;
// Main class named "test"
public class test {
// Main method
public static void main(String[] args) {
// Prompting the user to input the number (n)
System.out.println("Input the number(n):");
// Creating a Scanner object for user input
Scanner s = new Scanner(System.in);
// Reading the input number (n) from the user
int c = s.nextInt();
// Initializing a counter for prime numbers
int prime_ctr = 0;
// Loop to iterate through numbers and check for primes
for (int i = 2; i <= c; i++) {
// Checking if the current number is prime using the Check_Prime method
if (Check_Prime(i)) {
prime_ctr++;
}
}
// Displaying the number of prime numbers which are less than or equal to n
System.out.println("Number of prime numbers which are less than or equal to " + c + ": " + prime_ctr);
}
// Method to check if a given number is prime
public static boolean Check_Prime(int n) {
// Loop to check for factors of the number
for (int divisor = 2; divisor <= n / 2; divisor++) {
// If the number has a factor other than 1 and itself, it is not prime
if (n % divisor == 0) {
return false;
}
}
// If no factors were found, the number is prime
return true;
}
}
Sample Output:
Input the number(n): 1235 Number of prime numbers which are less than or equal to 1235: 202
Flowchart:
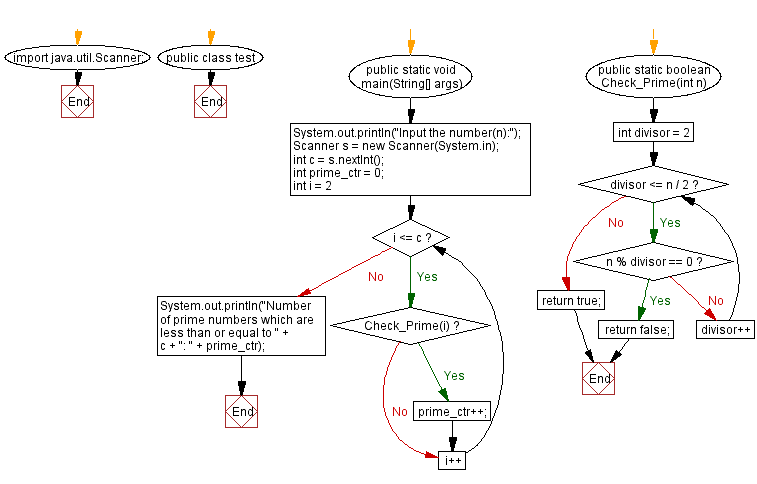
Java Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Java program which reads an integer n and find the number of combinations of a,b,c and d (0 ≤ a,b,c,d ≤ 9) where (a + b + c + d) will be equal to n.
Next: Write a Java program to compute the radius and the central coordinate (x, y) of a circle which is constructed by three given points on the plane surface.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/java-exercises/basic/java-basic-exercise-217.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics