Java: Compute the amount of the debt in n months
Compute Loan Debt After N Months
Write a Java program to compute the debt amount in n months. Monthly, the loan adds 4% interest to the $100,000 borrowed and rounds it to the nearest 1,000.
Input:
An integer n (0 ≤ n ≤ 100).
Sample Solution:
Java Code:
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
// Creating a Scanner object for user input
Scanner s = new Scanner(System.in);
// Prompting the user to input the number of months
System.out.println("Input number of months:");
// Reading the number of months from the user
int n = s.nextInt();
// Initializing the principal amount (initial debt) to 100,000
double c = 100000;
// Looping through each month to calculate the debt amount
for (int i = 0; i < n; i++) {
// Calculating the new debt amount after adding 4% interest
c += c * 0.04;
// Checking if the debt amount is not a multiple of 1000
if (c % 1000 != 0) {
// Reducing the debt amount to the nearest multiple of 1000
c -= c % 1000;
// Adding 1000 to the debt amount
c += 1000;
}
}
// Printing the final debt amount without decimal places
System.out.println("\nAmount of debt: ");
System.out.printf("%.0f\n", c);
}
}
Sample Output:
Input number of months: 6 Amount of debt: 129000
Flowchart:
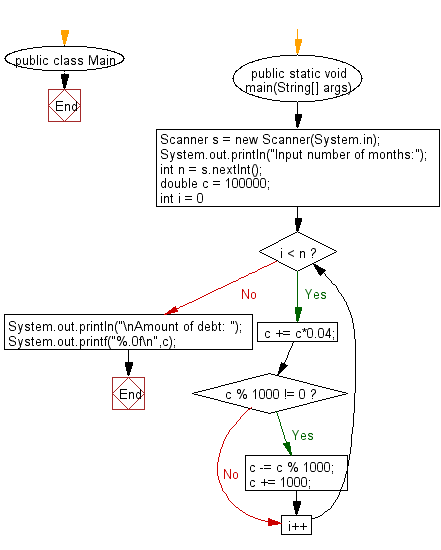
Java Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Java program which solve the equation. Print the values of x, y where a, b, c, d, e and f are specified.
Next: Write a Java program which reads an integer n and find the number of combinations of a,b,c and d will be equal to n.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics