Java: Check whether three given lengths of three sides form a right triangle
Check If Sides Form Right Triangle
Write a Java program to check whether three given lengths (integers) of three sides form a right triangle. Print "Yes" if the given sides form a right triangle otherwise print "No".
Input:
Integers separated by a single space. 1 ≤ length of the side ≤ 1,000
Visual Presentation:
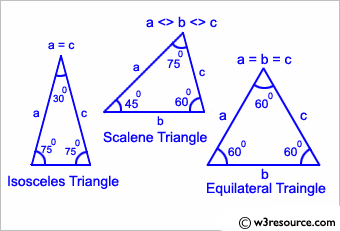
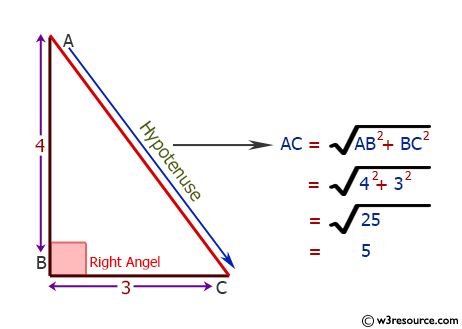
Sample Solution:
Java Code:
import java.util.Arrays;
import java.util.Comparator;
import java.util.Scanner;
class Main {
// Creating a Scanner object for user input
Scanner sc = new Scanner(System.in);
// Method to execute the main functionality
public void run() {
// Prompting the user to input three integers (sides of a triangle)
System.out.println("Input three integers(sides of a triangle)");
// Reading three integers and storing them in an array
int[] int_num = new int[]{
sc.nextInt(), sc.nextInt(), sc.nextInt()
};
// Sorting the array of integers in ascending order
Arrays.sort(int_num);
// Checking if the given sides form a right triangle
System.out.println("If the given sides form a right triangle?");
ln((int_num[2] * int_num[2] == int_num[0] * int_num[0] + int_num[1] * int_num[1]) ? "Yes" : "No");
}
// Main method to create an instance of the class and run the program
public static void main(String[] args) {
new Main().run();
}
// Method for printing without a newline
public static void pr(Object o) {
System.out.print(o);
}
// Method for printing with a newline
public static void ln(Object o) {
System.out.println(o);
}
// Method for printing an empty line
public static void ln() {
System.out.println();
}
}
Sample Output:
Input three integers(sides of a triangle) 6 9 12 If the given sides form a right triangle? No
Flowchart:
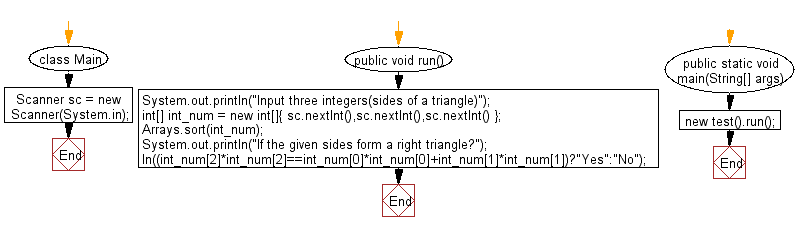
For more Practice: Solve these Related Problems:
- Write a Java program to check if three given side lengths form an acute, right, or obtuse triangle.
- Write a Java program to validate a right triangle using the Pythagorean theorem with floating-point precision.
- Write a Java program to classify a triangle by angles after confirming the sides form a right triangle.
- Write a Java program to check if three sides provided in random order can form a right triangle.
Java Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Java program to compute the digit number of sum of two given integers.
Next: Write a Java program which solve the equation. Print the values of x, y where a, b, c, d, e and f are specified
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics